Java, a stalwart in the programming world, balances simplicity with powerful features, allowing developers to craft robust, high-performance applications. Central to leveraging Java effectively are the concepts of variables and data literals. Each serves a distinct purpose in Java programming, facilitating the representation and manipulation of data in various forms.
Variables in Java are containers for storing data values that may change during the execution of a program, while data literals represent fixed values directly inserted into the code. Both are foundational to programming in Java, enabling developers to define the behavior and outcomes of their applications dynamically and with precision.
Understanding the difference between variables and data literals is crucial for developers aiming to optimize their Java applications. Variables offer flexibility and adaptability, allowing programs to process and store variable data efficiently. On the other hand, data literals provide a way to express constant values clearly and succinctly, enhancing code readability and maintainability.
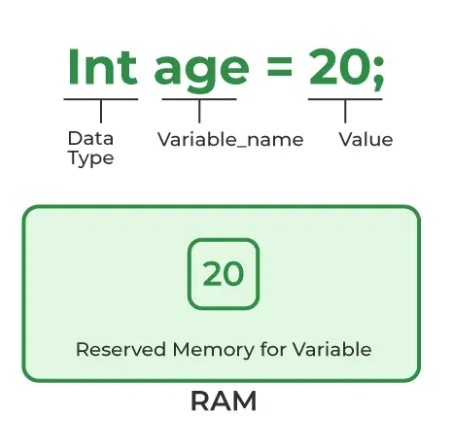
Variables in Java
Definition
In Java, variables are fundamental components that store data values within a program. These are not just placeholders but are essential for manipulating and accessing data as a program runs. Variables allow Java developers to write flexible and dynamic code, where the operations on data can adapt as the program executes.
Types
Primitive vs. Reference Variables
Java categorizes its variables into two main types: primitive and reference.
- Primitive variables store basic data types directly. These include
int
,char
,float
, andboolean
, among others. They hold values like numbers, single characters, or true/false conditions. - Reference variables, on the other hand, store the address of objects in memory rather than the data itself. They refer to instances of classes, including arrays and strings.
This distinction is crucial because it affects how data is handled and manipulated in your code.
Declaration
Declaring a variable in Java is straightforward. The syntax involves specifying the variable’s type followed by its name. Here’s the general structure:
bashCopy code
type variableName;
For example, to declare an integer variable named score
, you would write:
javaCopy code
int score;
Declaration does not automatically initialize the variable with a value; it simply tells the Java compiler that you’re reserving a space for a variable of a specific type.
Initialization
Initialization is the process of assigning a value to a declared variable for the first time. You can initialize a variable at the time of declaration or after it. Here are two ways to initialize the score
variable with a value of 10
:
- During Declaration:
javaCopy code
int score = 10;
- After Declaration:
javaCopy code
int score; score = 10;
Initialization is a critical step because it prepares the variable for use in operations and method calls within your program.
Data Literals in Java
Definition
Data literals in Java are fixed values that are written directly into the code. They represent immutable data that doesn’t change during the execution of the program. These literals are the raw values used to initialize variables or constants.
Types
Integer, Floating Point, Character, and String Literals
Java supports several types of literals, including:
- Integer Literals: These are used for numeric values without decimals. For example,
100
,200
, and-50
are integer literals. - Floating Point Literals: These represent decimal numbers or numbers in exponential form. For example,
3.14
,0.5
, and2E3
(which represents 2×10^3 or 2000) are floating point literals. - Character Literals: A single character surrounded by single quotes. For instance,
'A'
,'b'
, and'1'
are character literals. - String Literals: A sequence of characters surrounded by double quotes. For example,
"Hello, World!"
and"Java"
are string literals.
These literals are key to expressing constant values in a clear and efficient manner within your Java programs.
Usage
Examples of Data Literals in Code
Here are some examples demonstrating how data literals can be used in Java code:
- Initializing a variable with an integer literal:
javaCopy code
int age = 30;
- Using a floating point literal to represent a decimal value:
javaCopy code
double temperature = 36.5;
- Setting a character as a literal value:
javaCopy code
char grade = 'A';
- Defining a string with a literal:
javaCopy code
String greeting = "Hello, Java!";
Data literals make your code more readable and maintainable by allowing you to see at a glance the exact value being assigned or compared.
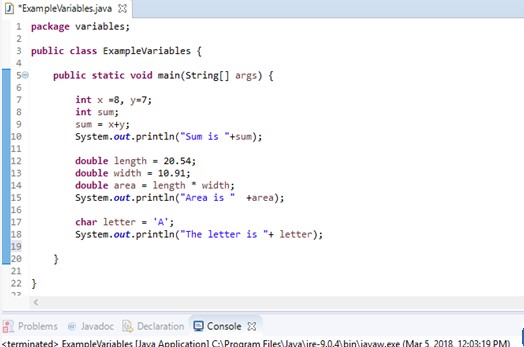
Comparing Variables and Data Literals
Nature
Mutable vs. Immutable
In Java, the nature of data handling divides into two categories: mutable (variables) and immutable (data literals).
- Variables are mutable, meaning their values can change over time. This is essential for dynamic data manipulation, allowing programs to adapt and modify data as needed.
- Data Literals are immutable; once they are set in the code, their values do not change. This immutability ensures consistency and predictability in parts of the code where fixed values are necessary.
Scope
Usage in Coding and Applications
- Variables find their usage across the board — from simple counter operations in loops to holding complex data structures in memory. They are pivotal in scenarios requiring data that evolves with the program’s execution, such as user input, file data processing, or real-time monitoring systems.
- Data Literals shine in situations where a constant value is crucial, such as configuration settings, constants (like Pi in mathematical operations), or for condition checks within the code. Their direct inclusion makes the code more readable and maintainable.
Memory Allocation
How Java Allocates Memory for Each
Java’s approach to memory allocation for variables and data literals varies significantly:
- Variables: Memory allocation for variables depends on their type. Primitive types are allocated memory in the stack, which allows for quick access but limited storage. Reference variables, pointing to objects, allocate memory in the heap, which is larger but slower to access.
- Data Literals: They are typically stored in the constant pool, a special area of the heap designed for storing canonicalized immutable objects, including literals. This makes retrieving these values efficient and reduces memory overhead by sharing common literals across the application.
Performance
Impact on Java Application Performance
- The mutable nature of variables means that Java applications can adapt and process data dynamically, enhancing functionality but requiring careful memory and resource management to maintain performance.
- Immutable data literals contribute to performance by reducing the need for memory allocation and making constant value access faster and more predictable. However, excessive use of literals, especially large strings or arrays, without proper consideration can lead to increased memory use.
Practical Applications
Variables
Use Cases in Real-world Programming
Variables are indispensable in real-world programming. Examples include:
- User Interaction: Storing user inputs for processing.
- Control Structures: Loop counters and condition flags.
- Data Storage: Temporarily holding data retrieved from databases.
- Dynamic Content Generation: Storing values that change over time, such as timestamps or randomized content.
Data Literals
Common Scenarios for Their Use
Data literals are commonly used for:
- Constants Definition: Defining fixed values such as
static final int MAX_SIZE = 100;
. - Configuration Values: Hard-coded settings within the code, like server URLs or API keys (though external configuration is often recommended).
- Conditional Logic: Embedding specific values directly into if-else or switch statements for clarity and direct comparison.
Best Practices
Variables
Guidelines for Effective Variable Use
To maximize the benefits of using variables, consider the following best practices:
- Clear Naming: Choose descriptive and meaningful names for variables to enhance code readability.
- Minimal Scope: Limit the scope of variables as much as possible to reduce memory usage and avoid unintended modifications.
- Initialization: Always initialize variables to avoid null pointer exceptions and unexpected behaviors.
- Type Appropriateness: Use the most specific and smallest data type needed to reduce memory consumption.
Data Literals
Tips for Using Data Literals Efficiently
When using data literals, keep these tips in mind:
- Avoid Magic Numbers: Use named constants instead of embedding literals directly in the code logic to make modifications easier and improve readability.
- String Pool Utilization: Reuse string literals to leverage Java’s string pool mechanism for memory efficiency.
- Final Variables: For constants, consider declaring variables as
final
along with literal assignments to combine the clarity of variable names with the immutability of literals.
FAQs
What are variables in Java?
Variables in Java are named memory locations that store data which can be modified during program execution. They are defined by a specific type that determines the size and layout of the memory storage, as well as the range of values that can be stored and the operations that can be performed on them.
How do data literals differ from variables?
Data literals in Java are fixed values explicitly written in the code. Unlike variables, which can change value throughout the execution of a program, data literals remain constant. They are used to represent values directly, making code easier to read and understand.
Why is understanding variables and data literals important?
Understanding variables and data literals is essential for effective Java programming. It enables developers to utilize data efficiently, allowing for the dynamic manipulation of values with variables and the clear expression of constants through literals. This understanding leads to more readable, maintainable, and efficient code.
Can data literals be used as variables?
Data literals cannot be used as variables; they are fixed values that do not change. However, they can be assigned to variables as initial values. This distinction allows programmers to use literals for defining constant values and variables for storing data that may change during program execution.
Conclusion
Grasping the distinction between variables and data literals is pivotal for any Java developer, as it underpins much of Java’s functionality and its application in real-world programming. Variables provide the flexibility to store and manipulate data dynamically, while data literals offer a means to embed immutable values directly into the code.
This understanding not only aids in writing clear and efficient code but also in appreciating the intricacies of Java’s design. By leveraging the strengths of both variables and data literals, developers can craft applications that are both powerful and efficient, truly harnessing the capabilities of Java to meet their programming needs.