In the realm of programming and software development, the efficiency and effectiveness of data handling significantly impact the performance and scalability of applications. A fundamental aspect of this data handling is the choice of data structures, specifically Lists and Sets, which serve different purposes and exhibit unique characteristics. Understanding the difference between these two data structures is crucial for developers to make informed decisions that align with their application’s needs and constraints.
The primary distinction between a List and a Set lies in their handling of data elements. Lists are ordered collections that can contain duplicate elements, allowing for indexing and retrieval based on position. On the other hand, Sets are unordered collections that do not allow duplicates, focusing on the uniqueness of each element. This fundamental difference dictates their respective use cases and operational efficiency in various scenarios.
Further exploring these data structures reveals their nuances, such as performance implications in searching, adding, and deleting elements, as well as their memory usage. Lists offer the advantage of preserving the order of elements and facilitating operations that require element sequencing. Sets, conversely, excel in situations where the primary concern is the presence or absence of unique elements, offering faster operations for checking the existence of items due to their inherent structure.
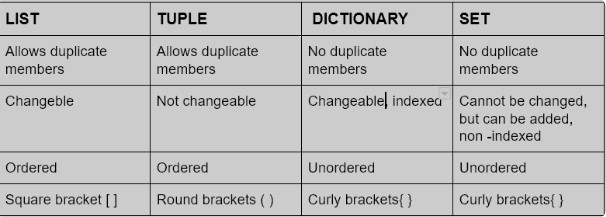
Core Concepts
Data Structures Overview
Data structures are fundamental concepts in computer science, crucial for storing, organizing, and managing data efficiently. Among the myriad of data structures available, Lists and Sets stand out for their versatility and wide range of applications. A List is an ordered collection of items that can include duplicates and maintain the order of insertion. On the other hand, a Set is a collection that is unordered and contains no duplicate elements. These properties make each suitable for different programming scenarios.
List Basics
A List is characterized by its ability to maintain an ordered sequence of elements, which can be of any type. Lists are dynamic, meaning their size can change during program execution. They support operations such as insertion, deletion, and iteration over the elements. Common uses of Lists include:
- Storing a collection of items where the order matters.
- Allowing duplicate values.
- Performing sequential operations, like searching for items in the order they were added.
Set Basics
A Set, in contrast to a List, is defined by its uniqueness and lack of order. Sets automatically remove duplicate entries and do not maintain the order of elements. This makes Sets ideal for:
- Ensuring a collection contains no duplicates.
- Fast membership testing, as Sets are optimized for checking whether an item is present.
- Operations like union, intersection, and difference, which are common in mathematical set theory.
Comparing List and Set
Order and Indexing
Lists maintain an order, allowing operations such as indexing, where you can access an element by its position. This property is crucial when the sequence of elements is important. Sets, however, do not support indexing due to their unordered nature. This difference highlights the suitability of Lists over Sets when the order of elements is critical.
Uniqueness
Uniqueness is a defining feature of Sets. They automatically eliminate duplicates, ensuring that each element is unique. This contrasts with Lists, which allow multiple occurrences of the same element. This property of Sets is particularly useful when you need to maintain a collection of distinct items.
Performance Implications
The structural differences between Lists and Sets have performance implications:
- Searching: Sets generally offer faster search operations than Lists because the underlying data structures are optimized for quick lookups.
- Addition and Deletion: Adding or removing elements in a Set is typically faster than in a List, especially for large datasets, due to the absence of order maintenance.
Memory Usage
Memory usage also varies between Lists and Sets. Lists can be more memory-efficient for small collections, especially if there are no duplicate elements. However, as the collection grows, the memory overhead of maintaining order in Lists can make Sets a more memory-efficient option.
Practical Examples
Example in Python
Python provides built-in support for both Lists and Sets, making it easy to utilize these structures.
Lists:
pythonCopy code
# Creating a list my_list = [1, 2, 2, 3, 4] # Accessing elements by index print(my_list[0]) # Output: 1 # Adding an element my_list.append(5) print(my_list) # Output: [1, 2, 2, 3, 4, 5]
Sets:
pythonCopy code
# Creating a set my_set = {1, 2, 3, 4} # Adding an element (no duplicates) my_set.add(2) print(my_set) # Output: {1, 2, 3, 4} # Adding a new element my_set.add(5) print(my_set) # Output: {1, 2, 3, 4, 5}
Example in Java
Java also offers robust implementations for Lists and Sets through its Collections Framework.
Lists:
javaCopy code
import java.util.ArrayList; import java.util.List; public class ListExample { public static void main(String[] args) { List<Integer> myList = new ArrayList<>(); myList.add(1); myList.add(2); myList.add(2); myList.add(3); System.out.println(myList.get(0)); // Output: 1 myList.add(4); System.out.println(myList); // Output: [1, 2, 2, 3, 4] } }
Sets:
javaCopy code
import java.util.HashSet; import java.util.Set; public class SetExample { public static void main(String[] args) { Set<Integer> mySet = new HashSet<>(); mySet.add(1); mySet.add(2); mySet.add(2); mySet.add(3); System.out.println(mySet); // Output: [1, 2, 3] mySet.add(4); System.out.println(mySet); // Output: [1, 2, 3, 4] } }
These examples in Python and Java illustrate the basic operations and behaviors of Lists and Sets, underscoring their differences and potential applications in software development.
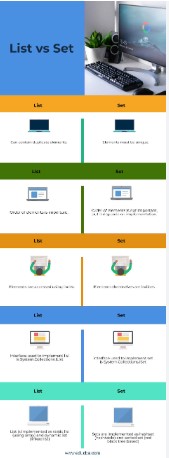
Use Case Scenarios
When to Use a List
Lists are the go-to data structure when order matters and you need to access elements by their position. They are ideal for:
- Maintaining Insertion Order: When the sequence in which elements are added is important, Lists preserve this order.
- Allowing Duplicates: In scenarios where you need to count occurrences or handle multiple instances of elements, Lists support this feature.
- Sequential Access: For operations that require access to elements in a specific sequence, Lists provide efficient support.
Examples include implementing a playlist for a media player where the order of songs matters or storing a series of user actions where duplicates may be necessary for repeated actions.
When to Use a Set
Sets are preferred when your primary concern is uniqueness of elements and you do not care about the order of items. Use Sets for:
- Ensuring Uniqueness: Automatically removing duplicates, Sets are perfect for collections of unique items like a user directory.
- Efficient Lookups: If you need to frequently check for the presence of an item without caring about its position, Sets offer faster lookups.
- Set Operations: For mathematical set operations like union, intersection, and difference, Sets are inherently designed to handle these efficiently.
A common use case is managing a collection of unique tags assigned to blog posts or articles, where each tag should appear only once.
Conversion Techniques
List to Set
Converting a List to a Set is straightforward and is commonly used to remove duplicates from a List. Here’s how you can do it in Python:
pythonCopy code
my_list = [1, 2, 2, 3, 4, 4, 5] my_set = set(my_list) print(my_set) # Output: {1, 2, 3, 4, 5}
In Java, the conversion looks like this:
javaCopy code
import java.util.*; public class Main { public static void main(String[] args) { List<Integer> myList = new ArrayList<>(Arrays.asList(1, 2, 2, 3, 4, 4, 5)); Set<Integer> mySet = new HashSet<>(myList); System.out.println(mySet); // Output: [1, 2, 3, 4, 5] } }
Set to List
Converting a Set to a List might be necessary when you need to order the elements after ensuring their uniqueness. In Python:
pythonCopy code
my_set = {1, 2, 3, 4, 5} my_list = list(my_set) print(my_list) # Output could vary due to set being unordered
For Java:
javaCopy code
import java.util.*; public class Main { public static void main(String[] args) { Set<Integer> mySet = new HashSet<>(Arrays.asList(1, 2, 3, 4, 5)); List<Integer> myList = new ArrayList<>(mySet); System.out.println(myList); // Output could vary } }
Advanced Considerations
Mutable vs Immutable
The concept of mutability vs immutability plays a crucial role in how Lists and Sets are handled across different programming languages. For instance, Python offers immutable versions of these structures, known as tuple
and frozenset
, which prevent modification after creation. This immutability can be essential for hashability and use as dictionary keys.
In contrast, Java Collections Framework does not provide immutable collections by default, but there are workarounds and libraries such as Collections.unmodifiableList
and Collections.unmodifiableSet
that offer read-only views.
Thread Safety
Thread safety is a critical consideration when using Lists and Sets in multi-threaded environments. Neither structure is inherently thread-safe in languages like Python and Java. However, both languages offer thread-safe versions or the means to make them thread-safe.
- Python: Use the
queue.Queue
for thread-safe operations in lieu of Lists. - Java: Collections like
Vector
andCopyOnWriteArrayList
for Lists, andConcurrentHashMap.KeySetView
for Sets provide thread-safe alternatives.
Frequently Asked Questions
Can I convert a List to a Set?
Yes, converting a List to a Set is a common operation in programming to remove duplicates and ensure the uniqueness of elements. This process typically involves passing the List to the constructor of a Set, which automatically filters out duplicate entries, leaving a collection of unique elements. The specific syntax and method might vary slightly across different programming languages.
Are Sets always faster than Lists?
While Sets generally offer faster performance for checking the presence of an element due to their unique structure, this does not mean Sets are always faster than Lists in every operation. Lists have better performance for indexed access and operations that benefit from order. The choice between using a Set or a List should be based on the specific needs of the application, such as whether order or uniqueness is more important.
How does order impact the use of Lists and Sets?
Order significantly impacts the choice between using a List or a Set. Lists maintain the order of elements, making them ideal for scenarios where sequence matters, such as maintaining a list of instructions or events in chronological order. Sets, being unordered, are preferable in situations where the arrangement of elements is irrelevant, and the focus is on ensuring element uniqueness and rapid checks for the presence of items.
Conclusion
The distinction between Lists and Sets is a fundamental concept in programming that directly influences the design and performance of applications. This article has elucidated the key differences, practical use cases, and considerations for choosing between these two data structures. It underscores the importance of understanding their characteristics to leverage each one’s strengths based on the specific requirements of a project.
Embracing the right data structure not only optimizes the efficiency of data operations but also contributes to the robustness and scalability of applications. Developers are encouraged to evaluate their needs carefully, considering factors like order, uniqueness, and performance, to select the most appropriate data structure. Experimentation and practical application further enrich this understanding, fostering more informed and effective programming practices.