Function prototypes and function definitions are fundamental components of C programming, each playing a crucial role in the creation of efficient and error-free code. While both are pivotal in the way functions are utilized and executed within a program, they serve distinct purposes and follow different syntax rules. Understanding the difference between these two elements is essential for any programmer looking to master C programming.
A function prototype in C is a declaration of a function that specifies its name, return type, and its parameters (if any) without providing the actual body of the function. On the other hand, a function definition includes not only the declaration of the function but also the complete body of the function, detailing the specific actions the function performs. Essentially, the prototype outlines a promise of functionality, while the definition fulfills this promise with concrete implementation.
Knowing when and how to use function prototypes and definitions is key to structuring C programs for clarity, maintainability, and effective compiler operation. These components help manage and organize code, especially in large projects where functions are used across multiple files or require forward declarations to avoid compilation errors.
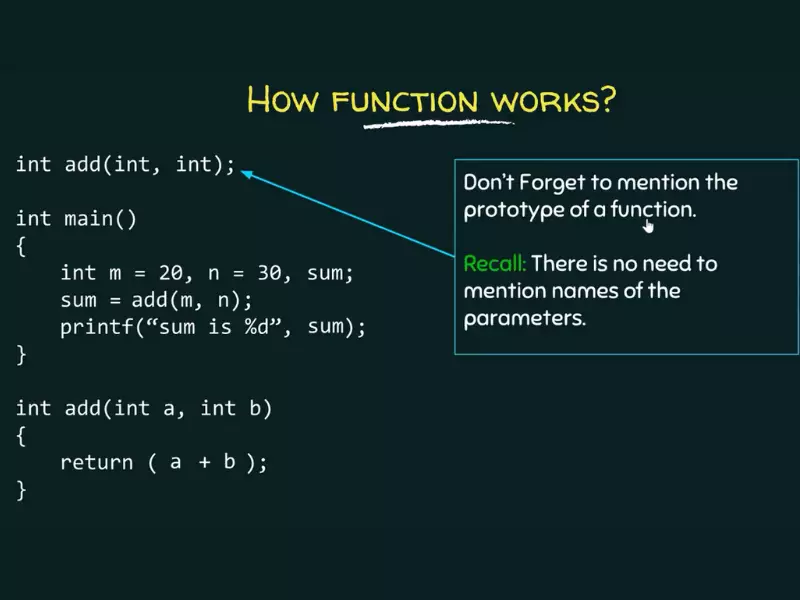
Function Basics
What are functions?
In C programming, a function is a block of code designed to perform a specific task. It is a fundamental building block of a program that allows for code reusability, modularity, and organization. A function can take inputs, process them, and return an output.
Functions help break down complex problems into smaller, manageable chunks. By defining a task in one place and calling the function as needed, you can avoid redundancy and make your code easier to read and maintain.
Role in C Programming
Functions play a critical role in C programming. They enable programmers to:
- Divide and Conquer: Break complex problems into smaller, more manageable tasks.
- Reuse Code: Write a function once and use it multiple times, reducing errors and saving time.
- Improve Readability: Make the code more organized and easier to understand.
- Facilitate Testing: Test small parts of the program independently for bugs.
Function Prototype
Definition
A function prototype is a declaration of a function that specifies the function’s name, return type, and parameters (if any), but not its body. It acts as a forward declaration, telling the compiler what a function looks like before it is used.
Purpose
The purpose of a function prototype is to:
- Inform the compiler about the function’s return type and parameter types before its actual definition.
- Enable function calls before the function is defined in the code.
Syntax Examples
cCopy code
int add(int, int); // Prototype for a function that adds two integers void printHello(); // Prototype for a function that prints "Hello" and returns nothing
Benefits
Function prototypes offer several benefits:
- Type Checking: They allow the compiler to perform type checks on function calls, reducing runtime errors.
- Code Organization: Enable better organization of code, especially in multi-file projects.
- Forward Declaration: Allow the use of functions before they are defined in the source code.
Function Definition
Definition
A function definition provides the actual body of the function. It includes the function’s name, return type, parameters, and the statements that define what the function does.
Purpose
The purpose of a function definition is to:
- Implement the functionality promised by the function prototype.
- Execute specific tasks and return a result when the function is called.
Syntax Examples
cCopy code
int add(int a, int b) { return a + b; // Function definition including the body } void printHello() { printf("Hello"); // Function definition including the body }
Components
A function definition consists of four main components:
- Return Type: The data type of the value the function returns.
- Function Name: The identifier for the function.
- Parameters: The inputs to the function, enclosed in parentheses.
- Function Body: The block of code that defines the function’s operations, enclosed in braces
{}
.
Key Differences
Syntax Distinction
The main syntax distinction between a function prototype and a function definition is that the prototype only declares the function’s interface, while the definition includes the actual code block.
Usage Context
- Function Prototypes are used when you need to inform the compiler about a function’s existence before its actual definition.
- Function Definitions are used to provide the complete functionality of the function.
Compilation Role
- Function Prototypes play a crucial role during the compilation process, allowing the compiler to check for correct function usage without needing the function’s body.
- Function Definitions are essential for the linking process, as they provide the code that must be executed when the function is called.
Parameter Necessity
- In function prototypes, it’s not mandatory to name the parameters; only their types are required.
- In function definitions, both parameter names and types are needed to implement the function’s logic.
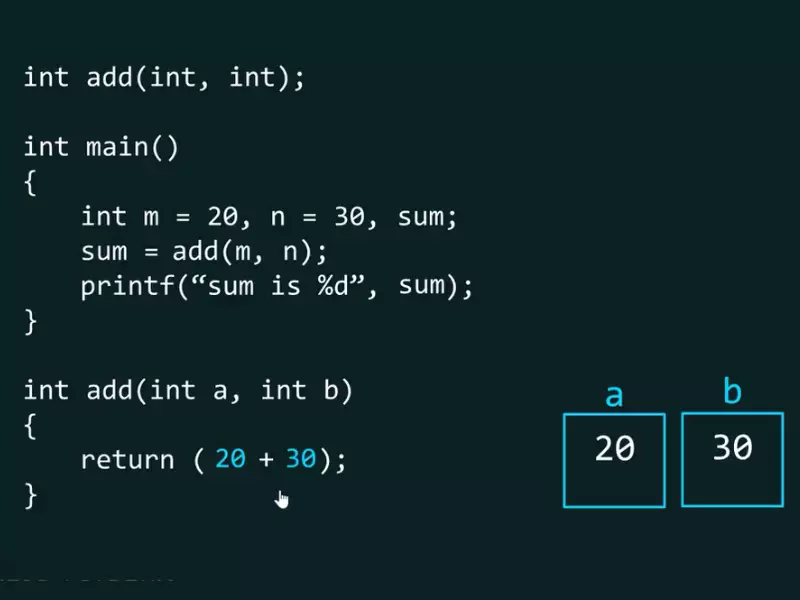
Prototypes vs. Definitions
When developing in C, understanding when to use function prototypes and function definitions is crucial for writing efficient, error-free code. Both serve important but distinct roles in the programming process.
When to Use Each
Function Prototypes are primarily used in the following scenarios:
- Before Function Definitions: When functions are called before they are defined in the code, prototypes prevent compiler errors by informing the compiler about the function’s existence and its signature.
- In Multi-File Projects: In projects spread across multiple files, prototypes in header files allow functions defined in one file to be used in others without having to include the entire definition.
Function Definitions are used to:
- Provide Functionality: Definitions are essential as they contain the actual code block that performs the operations specified by the function.
- Implement Logic: Once a function has been prototyped, its definition is required to implement the logic that was promised by the prototype.
Common Errors to Avoid
Several common errors can arise when working with function prototypes and definitions:
- Mismatched Signatures: Ensure the prototype and definition have exactly the same signature, including return type and parameter types.
- Omitted Prototypes: Forgetting to prototype a function before its first use can lead to compilation errors, especially in strict compilation modes.
- Duplicate Definitions: Defining a function more than once can cause linker errors. Ensure each function is defined exactly once across all files.
Best Practices
Adhering to best practices for using function prototypes and definitions can greatly enhance the readability, maintainability, and functionality of your C programs.
Prototyping Effectively
To prototype effectively, consider the following guidelines:
- Use Header Files: Place function prototypes in header files (.h) and include these headers in your C files (.c) where the functions are used or defined. This keeps prototypes organized and accessible.
- Keep Prototypes Consistent: Ensure that the prototype and the definition of the function have consistent signatures to prevent compilation errors.
- Document Prototypes: Comment your prototypes to indicate what the function does, what each parameter represents, and what the return value is. This improves code readability and helps other developers understand your code quickly.
Example:
cCopy code
// add.h #ifndef ADD_H // Prevent multiple inclusions #define ADD_H // Adds two integers and returns the result int add(int a, int b); #endif
Organizing Definitions
Organizing function definitions effectively is key to maintaining a clean codebase:
- Logical Grouping: Group related functions together in the same file or in closely related files. For example, all file operation functions could be placed in a file_operations.c file.
- Order of Definition: Define functions in an order that makes sense logically and for readability. Commonly called functions should be placed near the top of the file, and helper functions can be defined below them or in separate files.
- Use of Comments: Commenting your function definitions to explain complex logic or important details can help future maintainers understand the purpose and function of your code more easily.
Example:
cCopy code
// add.c #include "add.h" // Adds two integers and returns the result int add(int a, int b) { return a + b; }
Step-by-Step Guide for Organizing Code:
- Identify Related Functions: Determine which functions are related in functionality and group them together.
- Create Header Files: For each group of related functions, create a header file that contains their prototypes.
- Implement Definitions: Write the function definitions in .c files, ensuring they match the prototypes in your headers.
- Use Comments: Add comments to both your prototypes and definitions to explain their purpose, parameters, and return values.
- Review and Refactor: Periodically review your code organization to find opportunities to improve readability and maintainability. Refactor as necessary.
Frequently Asked Questions
What is a function prototype?
A function prototype is a declaration of a function that informs the compiler about the function name, its return type, and parameters without including the body of the function. It acts as a forward declaration, allowing the compiler to ensure that functions are called with the correct arguments across different parts of a program, enhancing code readability and maintainability.
Why is a function prototype necessary?
Function prototypes are necessary to provide early information to the compiler about function calls, enabling type checking of function arguments before the function is defined. This mechanism helps catch errors during the compilation process, ensuring that functions are used correctly and consistently throughout a program, thereby reducing runtime errors.
How does a function definition differ from a prototype?
A function definition differs from a prototype in that it includes the complete body of the function, detailing the specific actions that the function will perform. While a prototype acts as a declaration and promise of functionality, the definition provides the actual implementation, enabling the program to execute the defined tasks when the function is called.
Can a program work without function prototypes?
Yes, a program can work without function prototypes if the function definitions are placed before any calls to these functions within a file. However, using function prototypes is considered a best practice, especially in larger programs or those with multiple source files, as it enhances code organization, readability, and prevents common compilation errors related to undefined functions.
Conclusion
Understanding the distinction between function prototypes and function definitions is foundational for proficient C programming. These elements not only contribute to the structural integrity of a program but also play a significant role in its compilation and execution. By clearly defining the purpose and correct usage of each, programmers can write more efficient, maintainable, and error-free code.
The mastery of when to employ a function prototype versus a function definition reflects a deeper understanding of C programming principles. It’s this nuanced knowledge that separates proficient programmers from novices, enabling them to tackle complex coding challenges with greater ease and confidence. Ensuring clarity in the declaration and implementation of functions paves the way for more robust and reliable software development.