Constructors and destructors stand as the silent linchpins of object-oriented programming, orchestrating the lifecycle of objects from their creation to their eventual destruction. While their operations run behind the scenes, their role is critical in managing resources efficiently and ensuring the robustness of software applications. By handling initialization and cleanup, they provide a framework within which objects operate safely and effectively.
The key difference between constructors and destructors lies in their purpose and timing. Constructors are special methods invoked at the creation of an object, tasked with initializing an object’s properties and setting up its initial state. Destructors, on the other hand, are called when an object is no longer needed, responsible for freeing up resources and performing any necessary cleanup before the object’s memory is reclaimed.
Constructors prepare the stage for an object’s performance, equipping it with the necessary settings and resources to function. Destructors then ensure the stage is cleared properly, removing any lingering resources to prevent memory leaks or other issues. This dynamic ensures that every object gets a clean start and a proper closure, critical for maintaining application stability and efficiency.

Core Concepts
Constructors Explained
Definition and Role
In the realm of object-oriented programming (OOP), a constructor is a special type of method that is automatically invoked when an object of a class is created. The primary role of a constructor is to initialize the new object with default or specific values. Constructors ensure that the object starts its life in a consistent state, ready for use.
Types of Constructors
There are mainly three types of constructors in OOP: default, parameterized, and copy constructors. Each plays a vital role in initializing objects in different scenarios.
Default Constructor
A default constructor is the simplest form of a constructor, which does not take any arguments. It assigns default values to the object’s properties. If a class does not explicitly include any constructor, most languages automatically provide a default constructor.
Parameterized Constructor
A parameterized constructor allows passing arguments to the constructor, enabling the initialization of an object with specific values. This flexibility supports the creation of objects with distinct states right from their inception.
Copy Constructor
A copy constructor creates a new object as a copy of an existing object. This type of constructor is crucial for creating duplicate objects with the same properties and values as the original.
Destructors Demystified
Definition and Function
A destructor is another special method in OOP but works in contrast to a constructor. Its main function is to release resources that the object may have acquired during its lifecycle. Destructors are called automatically when an object is destroyed or goes out of scope, ensuring clean up and efficient resource management.
When Destructors are Called
Destructors are invoked automatically by the programming language’s runtime system when an object is no longer needed, typically when it goes out of scope or is explicitly deleted.
Key Differences
Purpose and Function
- Constructors prepare an object for use by initializing it.
- Destructors clean up resources before the object is destroyed.
Invocation Timing
- A constructor is called at the moment of object creation.
- A destructor is invoked just before an object is destroyed.
Parameter Handling
- Constructors can accept parameters for flexible object initialization.
- Destructors do not accept parameters, as their role is to clean up.
Memory Management
- Constructors and destructors are central to efficient memory management in OOP. Constructors allocate memory, while destructors release it, preventing leaks.
Practical Applications
Constructors in Use
Initializing Objects
Constructors enable the initialization of objects with desired states and values, ensuring that each object starts its life cycle ready for action.
Overloading Constructors for Flexibility
Overloading constructors means creating multiple constructors with different parameters. This allows for the creation of objects in various states, enhancing the flexibility and usability of a class.
Destructors at Work
Releasing Resources
Destructors play a critical role in freeing up resources like memory, file handles, and network connections, which are no longer needed by the object.
Automatic Cleanup Examples
- Automatic memory deallocation: Ensuring that dynamically allocated memory is freed.
- Closing file streams: Automatically closing files that were opened by the object.
- Releasing network resources: Ensuring that any network connections opened by the object are properly closed.
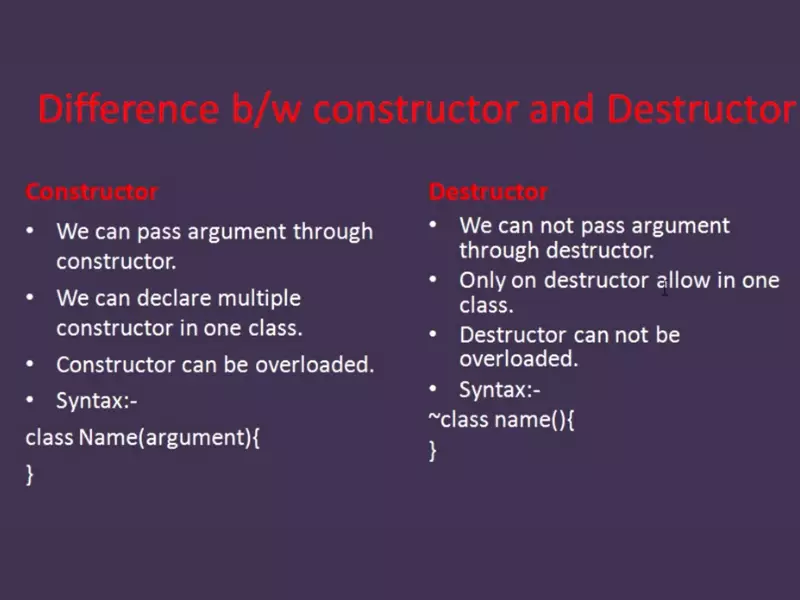
Language-Specific Perspectives
C++ Illustration
Syntax and Use Cases
C++ is a powerhouse for systems programming and game development, partly due to its control over system resources and complex functionalities. Constructors in C++ have a unique syntax that matches the class name and can be overloaded to serve various initialization purposes. Destructors, with a tilde (~) preceding the class name, ensure resources are released efficiently.
- Default Constructor: Automates object initialization.
- Parameterized Constructor: Allows passing values to objects at the time of creation.
- Copy Constructor: Enables copying of objects.
Destructors in C++ are called automatically when objects go out of scope or are explicitly deleted. Their primary use case is in dynamic memory allocation and the management of resources like file handles and network connections.
Java Context
Constructors and Garbage Collection
Java simplifies memory management through garbage collection, yet constructors play a vital role in setting up objects. Unlike C++, Java does not support destructors in the same way. Instead, it relies on a garbage collector to reclaim memory, which can run unpredictably. To manage resources efficiently, Java provides the finalize()
method, though its use is discouraged in favor of try-with-resources and explicit resource management.
- Constructors in Java are used to initialize objects.
- Garbage Collection automates memory cleanup, reducing the need for explicit destructors.
Advanced Insights
Impact on Software Design
The design and implementation of constructors and destructors significantly impact software design. They are crucial in many design patterns, such as Singleton, Factory Method, and Builder patterns, providing controlled access to the creation and destruction of objects.
- Singleton Pattern: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object but lets subclasses decide which class to instantiate.
- Builder Pattern: Separates the construction of a complex object from its representation.
Performance Considerations
The way constructors and destructors are implemented can greatly influence an application’s performance. Efficient use of these methods ensures minimal overhead during object creation and destruction, essential in performance-critical applications like real-time systems and high-frequency trading platforms.
- Optimization Tips:
- Minimize work in constructors and destructors.
- Avoid unnecessary object creation and destruction.
Common Pitfalls
Memory Leaks
One of the critical roles of destructors, especially in languages like C++, is to prevent memory leaks by ensuring that dynamically allocated resources are freed properly. Failure to correctly implement destructors can lead to memory not being released back to the system, causing the application to consume more memory over time and potentially crash.
- Best Practices: Always match every
new
with adelete
and everynew[]
with adelete[]
in C++ destructors.
Resource Management
Constructors and destructors are also pivotal in resource management strategies. Ensuring that resources such as file handles, network sockets, and database connections are properly acquired and released can prevent resource leaks and ensure that the application remains responsive and stable.
- Resource Acquisition Is Initialization (RAII): This C++ idiom ensures that resource allocation and deallocation are tied to object lifetime, automating resource management.
Frequently Asked Questions
Can a class have multiple constructors?
Yes, a class can have multiple constructors, each designed to initialize the object in different ways. This concept, known as constructor overloading, allows for the creation of objects with different initial states or properties, enhancing the flexibility and reusability of the class.
Is it necessary to explicitly define a destructor in a class?
It is not always necessary to explicitly define a destructor in a class. Most modern programming languages provide a default destructor that is automatically called to clean up basic resources. However, for classes that manage memory or other resources directly, defining a custom destructor is essential to ensure proper resource management and to prevent memory leaks.
How do constructors and destructors work in languages without explicit memory management?
In languages that handle memory management automatically, such as Java, constructors are still used for initializing objects, but destructors are replaced by garbage collection mechanisms. These mechanisms automatically reclaim memory when objects are no longer in use, reducing the need for explicit destructors but still requiring careful resource management within constructors.
Can destructors throw exceptions?
It is generally considered bad practice for destructors to throw exceptions. Throwing exceptions from destructors can lead to unforeseen behavior, especially during the cleanup phase of an object’s lifecycle. Instead, destructors should handle exceptions internally or ensure that all cleanup operations are exception-safe.
Conclusion
Constructors and destructors serve as fundamental components of object-oriented programming, each playing a pivotal role in managing the lifecycle of objects. Their effective use ensures not only the efficient allocation and deallocation of resources but also the overall stability and reliability of software applications. By providing a structured approach to initialization and cleanup, they encapsulate the complexities of resource management, making it easier for developers to create robust and efficient code.
The interplay between constructors and destructors underscores the importance of thoughtful software design, highlighting the need for careful consideration of how objects are created, used, and disposed of. In this light, understanding and leveraging these mechanisms to their full potential is essential for any developer looking to master object-oriented programming and develop high-quality software solutions.