Loops are fundamental components of programming, enabling developers to execute a block of code repeatedly under certain conditions. This iterative process is crucial for tasks that require repetitive execution, such as traversing arrays, processing data, or automating repetitive tasks. Two of the most commonly used looping constructs in various programming languages are the while
and do while
loops, each with its unique mechanism and use case.
The main difference between while
and do while
loops lies in their execution flow. A while
loop checks its condition at the beginning of each iteration, running the code block only if the condition evaluates to true. Conversely, a do while
loop executes its code block once before checking the condition at the end of the iteration, ensuring that the code block is executed at least once regardless of the condition’s truth value.
Understanding the nuances between while
and do while
loops is essential for making informed decisions about which loop to use based on the specific requirements of a programming task. The choice between these loops affects the code’s readability, efficiency, and overall execution flow, playing a significant role in the development of effective and error-free software.
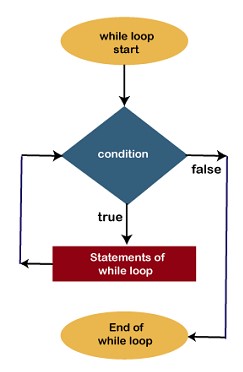
Loop Basics
What is a Loop?
A loop in programming is a fundamental concept used to execute a block of code repeatedly under certain conditions. This iterative process allows for the efficient execution of repetitive tasks such as data processing, automated testing, or any scenario where the same code needs to run multiple times. The purpose of a loop is to save time and effort, reducing the need for redundant code and making programs more efficient and easier to maintain.
Types of Loops
Programming languages typically offer several types of loops, each designed for specific scenarios and uses. The most commonly used loops are:
- For Loop: Ideal for when the number of iterations is known before the loop starts.
- While Loop: Best used when the number of iterations is not known and depends on a condition being met.
- Do While Loop: Similar to the while loop, but guarantees at least one iteration, even if the condition is false from the start.
- For Each Loop: Used to iterate over elements in a collection or array.
While Loop
Core Concept
The while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The loop begins with the condition check; if the condition is true, the code inside the loop is executed. This process repeats until the condition becomes false.
Explanation and Use Case
While loops are particularly useful in scenarios where the exact number of iterations is unknown before the loop begins. They are ideal for reading data until the end is reached, such as processing user input or reading a file line by line.
Syntax and Structure
Writing a while loop involves a simple syntax:
plaintextCopy code
while (condition) { // Code to execute }
- Start with the keyword
while
. - Follow it with a condition in parentheses.
- Write the code block to execute in curly braces.
Key Features
- Pre-Test: The condition is checked before executing the loop’s body.
- Flexibility: Can handle situations where the number of iterations is not known ahead of time.
- Risk of Infinite Loop: If the condition never becomes false, the loop will run indefinitely.
Example
A simple example of a while loop that prints numbers from 1 to 5:
pythonCopy code
i = 1 while i <= 5: print(i) i += 1
Do While Loop
Core Concept
The do while loop differs from the while loop by ensuring that the code block is executed at least once before the condition is checked. After the first execution, it behaves like a while loop, repeating the code block as long as the condition remains true.
Explanation and Use Case
Do while loops are useful in situations where the code block must be executed at least once, such as displaying a menu in a user interface where at least one interaction with the user is guaranteed.
Syntax and Structure
The basic structure of a do while loop is slightly different and might vary by programming language since not all languages directly support it. In languages like C, it looks like this:
plaintextCopy code
do { // Code to execute } while (condition);
- Start with the
do
keyword. - Write the code block to execute.
- Follow it with
while
, and the condition in parentheses, ending with a semicolon.
Key Features
- Post-Test: The loop executes once before the condition is checked.
- Guaranteed Execution: Ensures the loop’s body is executed at least once.
- Syntax Variation: Not all programming languages support do while loops natively.
Example
An example of a do while loop that prompts the user for input at least once:
cCopy code
#include <stdio.h> int main() { char continueLoop; do { printf("Do you want to continue? (y/n): "); scanf(" %c", &continueLoop); } while (continueLoop == 'y'); return 0; }
In this segment, we’ve explored the basics of loops, focusing on the while and do while loops. Through examples and explanations of their syntax and key features, we can appreciate their role in making programming more efficient and effective. Whether for iterating over data structures, processing user input, or automating repetitive tasks, understanding these loops is essential for any developer.
Comparative Analysis
Execution Flow
The fundamental difference between while loops and do while loops is their execution flow. While loops check their condition before the first iteration, making them entry-controlled. This means if the initial condition fails, the code block inside the while loop won’t execute even once. In contrast, do while loops execute their code block once before checking the condition, making them exit-controlled. This ensures that the code block inside a do while loop is executed at least once, regardless of whether the condition is true or false.
Entry vs. Exit Condition
- Entry-Controlled Loops (While Loop): The condition is evaluated before the execution of the loop’s body. If the condition is false at the first iteration, the loop’s body does not execute at all.
- Exit-Controlled Loops (Do While Loop): The condition is evaluated after the execution of the loop’s body, guaranteeing at least one execution of the loop’s body, regardless of the condition’s truth.
Use Cases
When to use each loop can often depend on the specific needs of the program:
- Use a while loop when you are unsure if the loop should run, as it checks the condition first.
- A do while loop is the better choice when the loop needs to run at least once, such as when asking for user input or displaying a menu to a user.
Syntax Differences
Key syntax distinctions include:
- While Loop: Starts with the
while
keyword followed by the condition in parentheses and then the code block. - Do While Loop: Begins with the
do
keyword followed by the code block and ends with thewhile
keyword and the condition in parentheses.
Pros and Cons
While Loop Advantages
Benefits of using while loops include:
- Flexibility in handling unknown iteration counts effectively.
- Prevents execution when conditions are not met from the outset, optimizing performance.
While Loop Disadvantages
Drawbacks include:
- Potential for infinite loops if not carefully managed.
- May not execute if the initial condition is false, which might not be desirable in all scenarios.
Do While Loop Advantages
Benefits include:
- Guaranteed execution of the loop body at least once, useful in certain user interaction scenarios.
- Post-test condition allows for a wider range of logical constructs.
Do While Loop Disadvantages
Drawbacks involve:
- Possible unnecessary execution once if the condition is never true after the first iteration.
- Slightly more complex syntax that may lead to errors if not used correctly.
Best Practices
Choosing the Right Loop
Factors to consider include:
- Nature of the condition: Entry or exit controlled.
- Necessity of execution: Whether the loop’s body needs to run at least once.
- Readability and maintainability of the code for future reference.
Common Mistakes
Pitfalls to avoid:
- Forgetting to update the loop variable: Leading to infinite loops.
- Misplacing the condition check, especially in do while loops, can lead to logical errors.
Real-world Applications
While Loops in Action
Examples from software development include:
- Reading files where the end of the file is not known in advance.
- Waiting for an event to occur, where it’s uncertain when the event will happen.
Do While Loops in Action
Examples include:
- User input validation where the prompt must be displayed at least once and repeated until valid input is received.
- Menu systems where the menu is shown to the user at least once, and the display continues based on user selections.
Frequently Asked Questions
When should I use a while loop over a do while loop?
Use a while
loop when you need the condition to be checked before the loop executes. This is ideal for scenarios where the loop may not need to run at all if the initial condition is not met. The while
loop ensures that the code block runs only when necessary, providing a safeguard against unnecessary executions.
Can a do while loop be replaced with a while loop?
Yes, a do while
loop can be replaced with a while
loop, but it requires careful adjustment of the loop’s structure and condition. To mimic a do while
loop’s behavior with a while
loop, the code block must be executed once before entering the loop, followed by the condition check at the end of each iteration. However, this might lead to more complex and less readable code, depending on the scenario.
Are there performance differences between while and do while loops?
The performance difference between while
and do while
loops is generally negligible in most scenarios. The choice between the two should be based more on the suitability of their execution flow for the task at hand rather than on performance concerns. However, in very large or performance-critical applications, the minimal overhead of the condition check’s positioning might be considered.
Conclusion
The distinction between while
and do while
loops is a fundamental concept in programming, reflecting the importance of control structures in dictating the flow of execution. By understanding the specific mechanics and applications of each loop, developers can write more efficient, readable, and maintainable code. The choice between these loops should be guided by the nature of the problem at hand, with a clear understanding of how each loop’s execution flow aligns with the task’s requirements.
Choosing the appropriate loop type is just one aspect of developing robust software. It highlights the broader importance of understanding the wide array of programming constructs available and their strategic application. As with any tool in a developer’s arsenal, the effectiveness of while
and do while
loops is maximized when used with discernment and in the appropriate context, underscoring the art and science that underpins software development.