Java’s robust architecture offers a comprehensive framework for managing errors and exceptions, pivotal in crafting resilient and stable applications. Among its arsenal for error handling, the throw
and throws
keywords play crucial roles, albeit serving distinct purposes. Their proper use not only ensures the smooth execution of applications but also aids in the debugging process, making them indispensable tools for Java developers.
The throw
keyword in Java is used to explicitly throw an exception from a method or any block of code, allowing developers to manually trigger specific error conditions. On the other hand, throws
is used in a method’s declaration to indicate that this method might throw exceptions, necessitating handling by the calling functions. This distinction is fundamental to understanding Java’s exception handling mechanism.
Exception handling in Java is a structured way to detect and manage runtime errors, preventing the abrupt termination of programs. It involves categorizing errors into different types of exceptions and employing mechanisms like try-catch
blocks to catch and deal with these exceptions effectively. The throw
and throws
keywords enrich this mechanism by providing the means to throw exceptions deliberately and declare potential exceptions, respectively.
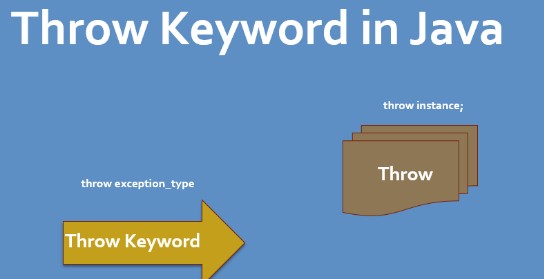
Java Exception Handling Basics
Definition of Exceptions
In Java, exceptions are events that disrupt the normal flow of a program’s instructions. These events are essentially objects that represent errors or issues that may occur during the execution of a program. When an error occurs within a method, it creates an exception object and hands it off to the runtime system. This process is known as throwing an exception. Exception handling is a powerful mechanism for controlling error handling in code, enabling developers to manage runtime errors effectively and maintain smooth program operation.
Exception Hierarchy
Java organizes exceptions in a hierarchy, making it easier to group and manage related exceptions. At the top of this hierarchy is the Throwable
class, which is divided into two main subclasses: Error
and Exception
. Errors are not meant to be caught by applications, as they are used by the Java runtime system to indicate serious problems that a reasonable application should not try to catch. Exceptions, on the other hand, are conditions that a program might want to catch.
The Exception
class has several subclasses, including:
- IOExceptions, which are thrown for failed or interrupted I/O operations.
- RuntimeExceptions, which represent problems that occur during the program’s runtime. These are unchecked exceptions, meaning they don’t need to be declared in a method’s
throws
clause.
Understanding this hierarchy helps in writing more precise and robust exception handling code.
Catching Exceptions
To manage exceptions effectively, Java provides a mechanism to catch and handle them. Catching an exception involves enclosing the code that might throw an exception within a try
block followed by one or more catch
blocks that handle the exception.
try
block: Contains the code that might throw an exception.catch
block: Catches and handles the exception.
Here’s a basic structure:
javaCopy code
try { // Code that might throw an exception } catch (ExceptionType name) { // Code to handle the exception }
Catching exceptions allows a program to continue executing, possibly taking corrective action, instead of crashing.
The throw
Keyword
Purpose
The throw
keyword in Java is used for manual exception throwing. It allows developers to create a new exception and hand it to the runtime, simulating the occurrence of an error condition. This capability is especially useful in custom error handling, where predefined exceptions might not sufficiently describe the problem.
Manual Exception Throwing
Manual exception throwing involves creating an instance of any subclass of the Throwable
class (typically an Exception
) and then using the throw
keyword to throw it. This action immediately stops the execution of the current method and hands the thrown exception to the runtime system for handling.
Usage
Using throw
is straightforward:
- Instantiate the exception object.
- Use
throw
to throw the exception.
Examples
Here’s a simple code snippet demonstrating the use of throw
:
javaCopy code
public void checkAge(int age) { if (age < 18) { throw new ArithmeticException("Access denied - You must be at least 18 years old."); } else { System.out.println("Access granted."); } }
The throws
Keyword
Purpose
While throw
is used to manually throw an exception, throws
is used in a method declaration to indicate that the method might throw exceptions. It’s a way of warning the caller of the method that they should handle the specified exceptions.
Method Exception Declaration
Using throws
in a method signature tells the Java compiler and runtime that this method might throw one or more exceptions, allowing for proper exception handling outside of the method.
Usage
To use throws
, include it in the method’s declaration followed by a list of the exception types the method can throw.
Examples
Here’s an example of using throws
in a method signature:
javaCopy code
public void readFile(String fileName) throws IOException { // Code that might throw an IOException }
In this example, the readFile
method declares that it might throw an IOException
, indicating to the method’s callers that they need to handle this type of exception.
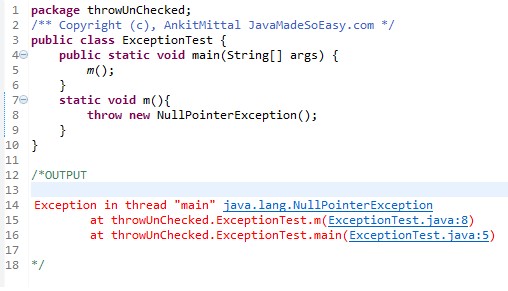
Key Differences
Syntax
Comparing throw
vs. throws
Syntax
The syntax difference between throw
and throws
is fundamental yet impactful. throw
is used inside a method to actually throw an exception, indicating an error has occurred. It is followed by an instance of an exception class (e.g., new Exception()
). On the other hand, throws
is used in the method signature to declare that a method might throw an exception, alerting the method’s caller to handle the potential exception. It is followed by the exception class names that might be thrown.
Scope
Operation Scope of Both Keywords
The operation scope also sets throw
and throws
apart. throw
operates within the method’s body, actively initiating an exception. It directly affects the flow of the method’s execution the moment it is invoked. Conversely, throws
operates at the method declaration level, serving as a warning mechanism without affecting the method’s internal logic or flow. It informs the compiler and developers about the potential exceptions that need handling outside the method.
Control Flow
Impact on Program Execution
The control flow of a program is significantly influenced by how throw
and throws
are used. Using throw
interrupts the normal flow of execution within a method, immediately transferring control to the nearest enclosing catch
block that can handle the thrown exception. If not caught, the exception propagates up the call stack. In contrast, throws
does not affect the immediate flow of execution but requires the calling method to either catch the declared exceptions or declare them in its own throws
clause, affecting how developers structure error handling across methods.
Use Cases
When to Use throw
Specific Scenarios for throw
throw
is most effective in the following scenarios:
- Custom Error Conditions: When standard exceptions do not adequately describe an error,
throw
allows for custom exceptions tailored to the specific needs of your application. - Error Handling within Methods: Use
throw
for controlling the flow within a method when an error condition is met. - Validating Method Arguments:
throw
is ideal for checking the validity of arguments passed to a method and throwing an exception if they do not meet required criteria.
When to Use throws
Ideal Scenarios for throws
throws
is particularly useful in scenarios such as:
- Delegating Exception Handling: When a method is not responsible for handling certain exceptions,
throws
delegates this responsibility to the caller. - API and Library Development: In APIs or libraries,
throws
clearly communicates to developers what exceptions they must handle when using your methods. - Complex Operations Requiring External Resources: Operations that depend on external systems (like file IO or network connections) are prone to errors outside your control.
throws
can declare these potential exceptions.
Common Mistakes
Misusing throw
and throws
Misunderstanding and misapplying throw
and throws
can lead to code smells, unclear error handling, and runtime errors. A common mistake is using throw
without considering the impact on the control flow, leading to abrupt program termination if the exception is not properly caught. Conversely, misusing throws
by declaring a broad swath of exceptions in the method signature can push too much responsibility onto the caller, complicating error handling.
Examples of Incorrect Usage
An example of incorrect usage is throwing an exception with throw
in a method but not properly documenting this behavior for callers, leading to unexpected runtime exceptions. Similarly, declaring a method with throws Exception
forces callers to handle all exceptions, even those that should have been handled within the method itself.
Overusing throws
Declaration
The Pitfalls of Declaring Too Many Exceptions
Overusing throws
can make your API or library cumbersome to use. Declaring a wide range of exceptions for a single method places undue burden on the caller, potentially leading to catch blocks that are too generic or extensive try-catch
structures that obscure the program’s logic.
Best Practices
Using throw
Effectively
To use throw
effectively:
- Be Specific: Throw exceptions that are as specific as possible to the error condition.
- Document: Clearly document the exceptions your method can throw, providing guidance on why the exception might be thrown and how callers should handle it.
- Use Custom Exceptions: For application-specific errors, consider defining and throwing custom exception classes that extend
Exception
orRuntimeException
.
Handling throws
Declaration
Strategies for declaring exceptions in methods with throws
include:
- Declare Specific Exceptions: Avoid using broad exception classes in
throws
clauses. Specify the exceptions that are actually thrown by the method. - Handle Internally When Possible: Handle exceptions within the method when practical, using
throws
for exceptions that truly require caller intervention. - Documentation: Just as with
throw
, document the conditions under which each declared exception might be thrown, aiding callers in understanding and handling them.
Frequently Asked Questions
Can throw
and throws
be used interchangeably?
No, throw
and throws
serve different purposes in Java and cannot be used interchangeably. throw
is used within a method to manually throw an exception, whereas throws
is part of a method’s signature, indicating that the method may throw exceptions and that they must be handled or declared by the calling method.
How does throw
affect program execution?
Using throw
in Java immediately transfers the control flow from the point of exception throwing to the nearest enclosing try-catch
block capable of handling the thrown exception. If not caught, the exception propagates up the call stack, potentially leading to the program’s termination if unhandled.
Is it necessary to handle all exceptions declared with throws
?
Yes, in Java, if a method declares one or more exceptions using throws
, the calling method must either handle these exceptions with a try-catch
block or declare them in its own throws
clause. This requirement ensures that all potential exceptions are adequately addressed, enhancing the robustness of the application.
Can we throw multiple exceptions with a single throw
statement?
No, a single throw
statement can only throw one exception instance at a time. However, a method can declare the ability to throw multiple exceptions using throws
, and multiple throw
statements can be used within a method to throw different exceptions under various conditions.
Conclusion
In conclusion, understanding the difference between throw
and throws
in Java is fundamental for effective exception handling. These two mechanisms provide developers with powerful tools to indicate and manage errors within applications, contributing significantly to their stability and robustness. By precisely controlling how exceptions are thrown and declared, programmers can ensure that their applications handle errors gracefully, maintaining smooth operation and enhancing user experience.
Moreover, the correct application of throw
and throws
reflects a deeper comprehension of Java’s exception handling framework, a critical aspect of writing clean, maintainable, and reliable code. As developers navigate through Java’s extensive features for managing runtime errors, mastering these keywords becomes a testament to their proficiency and commitment to crafting high-quality software.