Java stands as a cornerstone in the realm of programming languages, renowned for its robust structure and object-oriented capabilities. At the heart of its functionality are two pivotal keywords: this
and super
. These keywords, though simple in appearance, play crucial roles in the nuanced landscape of Java programming, enabling clearer, more efficient code by distinguishing between current and parent class components.
The difference between this
and super
in Java lies primarily in their contextual usage. This
refers to the current instance of a class, allowing access to its methods, constructors, and fields. Conversely, super
pertains to the parent class, enabling the child class to access its superclass’s methods, constructors, and fields. This distinction is fundamental to implementing inheritance and achieving polymorphism in Java.
Understanding the precise application and implications of this
and super
is essential for Java developers. These keywords not only enhance code readability and maintainability but also prevent common programming errors such as variable shadowing and ambiguous method invocation. Through strategic use, programmers can effectively manage class hierarchies, ensuring that each class behaves and interacts as intended within the larger application structure.
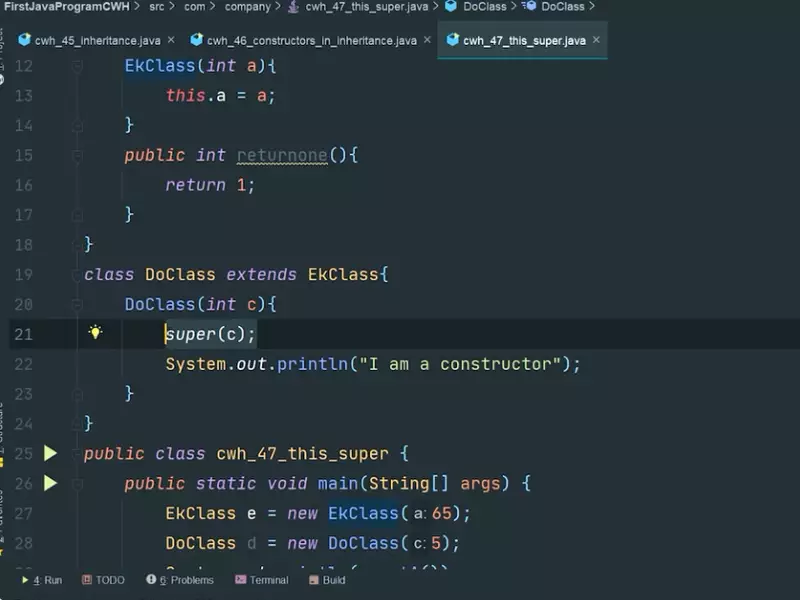
Java Basics
Key Concepts
Java, a high-level programming language, is centered around the principles of object-oriented programming (OOP). This paradigm emphasizes the concept of objects, which are instances of classes, designed to model real-world entities. OOP in Java is built on four fundamental concepts: encapsulation, inheritance, polymorphism, and abstraction.
- Encapsulation protects the data (attributes) and code (methods) from unauthorized access and prevents the data from being exposed to the outside world.
- Inheritance allows a new class to inherit properties and methods of an existing class, promoting code reusability.
- Polymorphism enables a single action to behave differently based on the object that is acting upon.
- Abstraction hides complex realities while exposing only the necessary parts.
Class Hierarchy
In Java, classes can be related to each other in a hierarchy, akin to a family tree. At the top is the Object class, the root of the class hierarchy, from which all other classes derive. This hierarchical structure is pivotal in Java’s inheritance mechanism, enabling a class to inherit fields and methods from its superclass. Classes that do not specify a superclass implicitly inherit from the Object class.
The this
Keyword
Definition
The this
keyword in Java is a reference variable that refers to the current object. It’s a way for an object to refer to itself.
Role in Java
This
plays a crucial role in avoiding naming conflicts between instance variables and parameters and is used within constructors, methods, and setters.
Usage
Accessing fields
When a field in a class shares the same name as a parameter in a method or constructor, this
helps distinguish the instance variable from the parameter. For example:
javaCopy code
public class Car { private String color; public Car(String color) { this.color = color; // Distinguishes the instance variable from the parameter } }
Invoking current class methods
This
can also be used to invoke other methods of the current class:
javaCopy code
public void setModel(String model) { this.setModel(model); }
Constructor chaining
Constructor chaining allows one constructor to call another within the same class using this
:
javaCopy code
public Car() { this("red"); // Calls another constructor in the same class }
The super
Keyword
Definition
Super
is a reference variable used to refer to the immediate parent class object.
Role in Java
It is primarily used to access methods and fields of the parent class, especially when subclass and parent class have methods and fields with the same name.
Usage
Accessing parent class fields
Super
allows a subclass to access fields of its parent class:
javaCopy code
public class Vehicle { protected String fuelType; } public class Car extends Vehicle { public void display() { super.fuelType = "Petrol"; System.out.println("Fuel Type: " + super.fuelType); } }
Invoking parent class methods
A subclass can use super
to call methods of the parent class that it overrides:
javaCopy code
@Override public void display() { super.display(); // Calls the display method of the parent class }
Constructor invocation
Super
can be used in a subclass constructor to invoke the constructor of its superclass:
javaCopy code
public Car() { super(); // Calls the constructor of the parent class, Vehicle }
Key Differences
Context Usage
this
vssuper
in accessing variables:this
is used to refer to the current class’s variables, whilesuper
refers to the parent class’s variables.
Method Invocation
- Calling current vs parent class methods:
this.methodName()
calls a method in the current class, whereassuper.methodName()
calls a method from the parent class.
Constructors
- How they differ in constructor chaining:
this()
is used for calling another constructor in the same class, whilesuper()
is used for calling a superclass’s constructor.
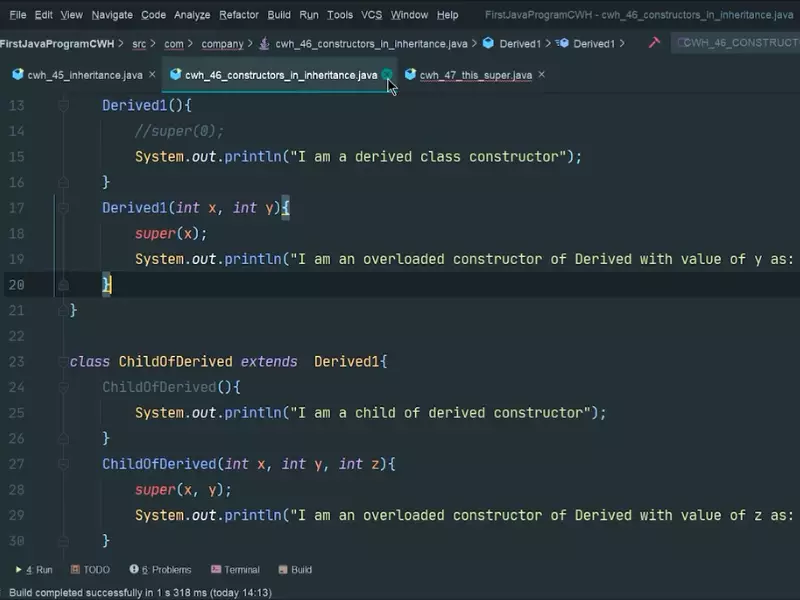
Practical Examples
Field Access
Comparing this
and super
in fields
Using this
and super
to access fields helps clarify which variables you’re working with, especially when dealing with inheritance and variable shadowing. Let’s dive into examples to see how they differentiate in accessing fields.
Example with this
:
javaCopy code
public class Person { String name = "John"; void printName() { String name = "Peter"; System.out.println(this.name); // Prints "John" } }
In this case, this.name
refers to the class field name
, not the local variable within printName()
method. It helps to distinguish between class fields and local variables when they have the same name.
Example with super
:
javaCopy code
public class Parent { String hobby = "Gardening"; } public class Child extends Parent { String hobby = "Painting"; void printParentHobby() { System.out.println(super.hobby); // Prints "Gardening" } }
Here, super.hobby
accesses the hobby
field of the Parent
class, not the Child
class, helping to avoid confusion between class variables shadowed by subclass variables.
Method Access
Demonstrating method override scenarios
Method overriding occurs when a subclass has a method with the same name, return type, and parameters as a method in its superclass. Let’s see how this
and super
can be used in such scenarios.
Overriding Example:
javaCopy code
class Animal { void eat() { System.out.println("Animal eats"); } } class Dog extends Animal { @Override void eat() { super.eat(); // Calls the eat method of Animal class System.out.println("Dog eats"); } } public class Test { public static void main(String[] args) { new Dog().eat(); // Output: // Animal eats // Dog eats } }
This demonstrates using super
to call the superclass method that has been overridden by the subclass. It’s useful for extending the functionality of a method from the superclass rather than completely replacing it.
Constructor Use
Examples of constructor chaining
Constructor chaining is the process of calling one constructor from another within the same class using this
, or calling a superclass constructor from a subclass using super
.
Constructor Chaining with this
:
javaCopy code
public class Rectangle { private int x, y; private int width, height; public Rectangle() { this(0, 0, 1, 1); } public Rectangle(int width, int height) { this(0, 0, width, height); } public Rectangle(int x, int y, int width, int height) { this.x = x; this.y = y; this.width = width; this.height = height; } }
This approach allows a class to have multiple constructors with different parameters, with one constructor calling another and reducing duplicated code.
Superclass Constructor Invocation with super
:
javaCopy code
public class Parent { Parent() { System.out.println("Parent Constructor"); } } public class Child extends Parent { Child() { super(); // Calls Parent's constructor System.out.println("Child Constructor"); } } public class Test { public static void main(String[] args) { new Child(); // Output: // Parent Constructor // Child Constructor } }
This example shows how super
is used to explicitly call a superclass’s constructor, ensuring that the initialization code in the superclass is executed.
Best Practices
When to Use this
- Variable Shadowing: Use
this
to resolve naming conflicts between instance variables and parameters in constructors or methods. - Constructor Chaining: Utilize
this
to call another constructor within the same class to avoid code duplication. - Clarity: Apply
this
for clarity when referring to the current object’s fields and methods, even without naming conflicts.
When to Use super
- Accessing Overridden Methods: Use
super
to call a method from the superclass that has been overridden in the current class. - Accessing Superclass Fields: Apply
super
when you need to access fields in the superclass that are hidden by subclass fields. - Superclass Constructor Calls: Invoke a superclass constructor using
super
at the beginning of a subclass constructor to ensure proper initialization.
Avoiding Common Pitfalls
- Misusing
this
andsuper
: Avoid usingthis
orsuper
where not necessary, as it can make the code more difficult to read and maintain. - Static Context: Remember, neither
this
norsuper
can be used in a static context, as they both refer to instances, not classes. - Constructor Overuse: Be mindful of overusing constructor chaining with
this
, as it can lead to complex and hard-to-follow initialization paths. - Superclass Method Overriding: When overriding methods, carefully decide whether to complement (using
super.methodName()
) or completely override the superclass method to avoid unintended behavior.
By adhering to these best practices, Java developers can effectively use this
and super
to write clear, concise, and maintainable code. Understanding when and how to use these keywords is crucial for navigating Java’s object-oriented features and avoiding common coding pitfalls.
FAQs
What is this
keyword in Java?
The this
keyword in Java is a reference variable that refers to the current object. It is used within an instance method or a constructor to refer to the current object on which the method or constructor is being invoked. This keyword helps to distinguish between class fields and parameters with the same name, and can also be used to invoke other constructors within the same class.
How does super
keyword work in Java?
The super
keyword in Java is used by a subclass to access methods and fields of its superclass. It serves as a reference to the parent class, allowing subclasses to access superclass methods that have been overridden, access superclass constructors, and reference fields of the superclass that may be hidden by fields in the subclass.
When should I use this
keyword?
Use the this
keyword when you need to refer to the current class instance. It is particularly useful in constructors and methods to distinguish between instance variables and parameters with the same name. Additionally, this
can be used to invoke other constructors within the same class in constructor chaining.
Can this
and super
be used in static contexts?
No, both this
and super
cannot be used in static contexts. Since this
refers to the current instance of a class and super
refers to the superclass instance, they do not make sense in a static context where an instance is not available. Static methods and fields belong to the class itself, not to any particular instance.
Conclusion
In the intricate dance of Java programming, this
and super
keywords emerge as fundamental steps, guiding the choreography of inheritance and class interaction. Their adept use marks the difference between code that is merely functional and code that is clear, efficient, and hierarchically well-organized. By distinguishing between current and parent class contexts, they empower developers to craft code with precision and intent.
Understanding and applying this
and super
effectively is a hallmark of experienced Java developers. It exemplifies a deeper comprehension of object-oriented programming principles and Java’s architectural nuances. As developers master these keywords, they unlock the potential for creating more sophisticated, robust Java applications. This knowledge not only enhances code quality but also enriches the developer’s toolkit, paving the way for advanced software development endeavors.