When embarking on the journey of programming, one quickly encounters various obstacles that can hinder the path to creating efficient and error-free code. Among these challenges, syntax and logical errors stand out as common culprits that can cause frustration and confusion. These errors, although different in nature, play a significant role in the debugging process, requiring a keen eye and a deep understanding to resolve effectively.
Syntax errors and logical errors are two fundamental types of errors in programming. A syntax error occurs when the code violates the rules of the programming language, making it impossible for the compiler or interpreter to understand and execute the code. On the other hand, a logical error is a mistake in the program’s logic that leads to incorrect output, even though the code is syntactically correct and can be executed.
Distinguishing between syntax and logical errors is crucial for developers, as each requires a different approach for identification and resolution. Syntax errors are often easier to detect and correct, thanks to the explicit feedback provided by compilers and interpreters. In contrast, logical errors can be elusive, hiding within the seemingly correct code and manifesting through unexpected behavior or incorrect output, challenging programmers to think critically about their code’s logic and intended outcomes.

Syntax Errors Defined
Explanation of Syntax Errors
In the world of programming, syntax errors are like the grammatical mistakes in our spoken languages. Just as a misplaced comma or a wrong verb tense can change the meaning of a sentence, syntax errors in coding disrupt the communication between the programmer and the computer. A syntax error represents a breach of the programming language’s rules, making the code unreadable to the compiler or interpreter. This type of error is fundamental and must be resolved for the code to execute.
Common Causes
Syntax errors can arise from a variety of simple oversights:
- Misspelling keywords or functions names
- Incorrect use of punctuation (e.g., missing semicolons or misused brackets)
- Wrong ordering of code elements
- Mismatched parentheses, brackets, or braces
These mistakes are often easy to make, especially for beginners or when working with unfamiliar languages.
Examples and Resolution
Consider the following examples of syntax errors and their solutions:
- Misspelled Keyword:
- Error:
pritn("Hello, world!")
- Resolution: Correct the spelling to
print("Hello, world!")
- Error:
- Missing Semicolon:
- Error (in languages like Java or C):
int x = 5
- Resolution: Add a semicolon
int x = 5;
- Error (in languages like Java or C):
- Mismatched Brackets:
- Error:
if (x > 5 { print(x) }
- Resolution: Correct the brackets to
if (x > 5) { print(x); }
- Error:
Correcting syntax errors often involves carefully reviewing error messages and code lines indicated by the development environment, ensuring adherence to the language’s syntax rules.
Logical Errors Explained
Understanding Logical Errors
Logical errors are the tricksters of programming errors. They occur when a program runs without crashing but produces incorrect results due to flaws in its logic. These errors reflect a misunderstanding or mistake in the algorithm’s design, leading to outcomes that deviate from the intended behavior. Unlike syntax errors, logical errors do not prevent the code from executing, making them significantly harder to detect and fix.
Typical Logical Error Scenarios
Logical errors can manifest in various ways, including:
- Incorrect calculations due to wrong formulas or operations
- Failing to account for certain conditions in the code
- Improperly ordered instructions leading to unintended consequences
These scenarios often result from overlooking the detailed requirements of the programming task or misinterpreting how certain functions or statements work.
Detection and Troubleshooting
Finding and fixing logical errors involves a few key strategies:
- Test extensively: Use a wide range of test cases to cover all possible scenarios.
- Debug step-by-step: Employ debugging tools to trace the execution flow and inspect variables.
- Peer review: Have another programmer review the code, as they might spot mistakes you’ve missed.
Identifying logical errors requires patience, a thorough understanding of the intended program logic, and sometimes a bit of creativity.
Key Differences
Syntax vs. Logic: A Comparative Analysis
The primary distinction between syntax and logical errors lies in their nature and impact on the code execution. Syntax errors are structural, preventing a program from running until they are corrected. They are easily identified by compilers or interpreters, which provide specific error messages pointing to the location and nature of the mistake.
On the other hand, logical errors are conceptual, affecting the program’s output or behavior rather than its ability to run. These errors slip past compilers unnoticed because the code is structurally sound; it simply doesn’t do what it’s supposed to do. Detecting logical errors requires a good understanding of the program’s intended outcome and meticulous testing.
Impact on Program Execution
- Syntax errors halt program execution, making them immediately apparent but typically easy to fix once identified.
- Logical errors allow the program to run but lead to incorrect results or behavior, making them harder to diagnose and correct.
Error Identification Strategies
Effective error identification is crucial for efficient programming. For syntax errors, programmers rely heavily on the development environment’s error messages. These messages are designed to be precise, often indicating the exact line and nature of the error.
To identify logical errors, programmers use a variety of techniques, including:
- Code reviews: Sharing code with peers can uncover errors that one might not notice alone.
- Unit testing: Writing tests for individual components can help ensure they work as expected in isolation.
- Integration testing: Testing combined components can detect errors in the interaction between different parts of the program.
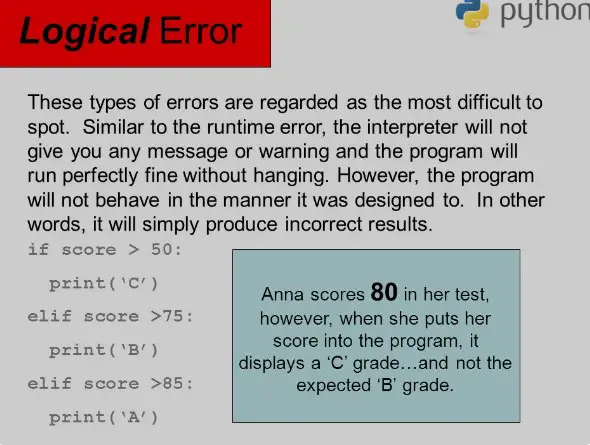
Error Resolution Techniques
Developing software is inherently challenging, and encountering errors is a natural part of the process. However, the ability to effectively resolve these errors is what sets apart successful projects. Let’s explore some advanced strategies and tools designed for tackling syntax and logical errors, along with best practices for debugging.
Tools and Techniques for Syntax Error Detection
Syntax errors, being the more straightforward to identify and resolve, benefit greatly from a variety of tools and techniques available to developers:
- Integrated Development Environments (IDEs): Modern IDEs are equipped with syntax highlighting, code completion, and real-time error detection features that can significantly reduce the incidence of syntax errors.
- Linters: Linters are tools that statically analyze your code for errors and coding standards. They can catch syntax errors before the code is compiled or executed.
- Compiler Error Messages: Paying close attention to compiler error messages is crucial. They often provide the exact location and nature of the syntax error, making it easier to correct.
Strategies for Identifying Logical Errors
Identifying logical errors requires a more nuanced approach, as these errors do not prevent code execution but lead to incorrect outcomes. Here are some strategies to uncover and resolve logical errors:
- Code Walkthroughs: Manually going through the code line by line can help understand the logic flow and spot discrepancies.
- Debugging Tools: Utilize debugging tools that allow you to step through the code execution, inspect variables, and monitor the program flow in real-time.
- Automated Testing: Implementing unit tests and integration tests can help catch logical errors by validating the code’s output against expected results.
Best Practices for Debugging
Debugging is both an art and a science, and adopting best practices can make the process more efficient:
- Start Simple: Begin with simple test cases and progressively move to more complex scenarios to isolate the error.
- Keep Changes Small: Make small, incremental changes and test frequently. This helps in identifying which changes fixed or did not fix the error.
- Use Version Control: Leverage version control systems to track changes and revert to previous states if a new bug is introduced during the debugging process.
Case Studies
Real-world examples serve as excellent learning tools. Here are a few case studies highlighting syntax and logical errors in actual programming scenarios.
Real-World Examples of Syntax Errors
Example 1: A developer forgot to close a string literal in a JavaScript code, leading to a syntax error. The code console.log("Hello, world!);
resulted in an error because the closing double-quote was missing.
Resolution: Adding the missing double-quote fixed the error: console.log("Hello, world!");
Analyzing Logical Error Scenarios
Example 2: In a Python program designed to calculate the average of a list of numbers, the developer mistakenly divided the sum of the numbers by the number itself instead of the list’s length. This logical error produced incorrect results.
Resolution: The developer corrected the code to divide by the length of the list, resolving the logical error and producing the correct average calculation.
Preventative Measures
Preventing errors before they occur is always preferable to fixing them afterwards. Here are some proactive coding practices and strategies to minimize syntax and logical errors.
Coding Practices to Avoid Syntax Errors
To minimize syntax errors, developers can:
- Follow Naming Conventions: Consistently use naming conventions to avoid confusion and typos.
- Use Code Snippets: Leverage code snippets for repetitive code patterns to reduce manual coding errors.
- Regular Code Reviews: Engage in code reviews with peers to catch errors early in the development process.
Logic Error Minimization Strategies
Minimizing logical errors requires a deep understanding of the problem being solved and the logic applied. Some strategies include:
- Clear Problem Definition: Ensure you have a thorough understanding of the problem and the expected outcome before starting to code.
- Pseudocode: Writing pseudocode can help plan the logic of the program before implementing it in code, reducing the likelihood of logical errors.
- Incremental Development: Build and test the program in small, manageable increments, verifying the logic at each step.

Frequently Asked Questions
What is a syntax error in programming?
A syntax error in programming refers to a violation of the grammatical rules of a programming language. It occurs when a programmer writes code that the compiler or interpreter cannot understand, often due to misspelled keywords, missing punctuation, or incorrect use of syntax. These errors prevent the code from compiling or running and must be corrected for the program to function.
How do logical errors differ from syntax errors?
Logical errors differ from syntax errors in that they occur when the program runs without crashing, but it produces incorrect results. These errors are due to mistakes in the program’s logic, where the written code does not perform the intended actions or calculations. Detecting logical errors requires thorough testing and debugging, as they do not produce explicit error messages like syntax errors.
Can syntax errors be automatically detected?
Yes, syntax errors can be automatically detected by compilers or interpreters during the compilation or interpretation process. These tools analyze the code against the programming language’s syntax rules and generate error messages indicating the nature and location of the syntax violations. This immediate feedback allows programmers to correct syntax errors before running the program.
Why are logical errors harder to detect?
Logical errors are harder to detect because the code is syntactically correct, so it compiles and runs without triggering error messages. Identifying logical errors requires understanding the intended functionality of the program and analyzing its actual output. Developers must often rely on debugging tools, test cases, and a systematic review of the code to uncover and resolve logical errors.
Conclusion
Understanding the distinction between syntax and logical errors is a cornerstone of programming proficiency. Syntax errors, with their straightforward identification and correction process, offer a clear path to resolving issues that prevent code execution. In contrast, logical errors demand a deeper insight into the program’s intended behavior, challenging developers to refine their debugging skills and attention to detail.
The ability to efficiently diagnose and fix these errors not only enhances code quality but also fosters a more intuitive understanding of programming languages and logic. Armed with the knowledge and strategies to tackle both syntax and logical errors, programmers can navigate the complexities of code development with confidence, ultimately crafting robust, error-free applications that perform as intended.