Spring and Hibernate stand as two pillars in the world of Java development, each offering unique features and capabilities to developers. While Spring is a comprehensive framework that provides infrastructure support for developing Java applications, Hibernate specializes in data persistence, making the database interactions seamless and efficient. The synergy and distinctions between these two technologies significantly influence the architecture and functionality of Java applications.
The primary difference between Spring and Hibernate lies in their core functionality and application within the Java ecosystem. Spring is a versatile framework designed to address and streamline the full spectrum of application development needs, from security to web applications. Hibernate, on the other hand, is an Object-Relational Mapping (ORM) tool that excels at facilitating the mapping of an application’s domain objects to the database tables. It simplifies data persistence and retrieval tasks, making it invaluable for database operations.
Both frameworks aim to reduce the complexity of development and improve productivity. Spring offers a robust set of features for comprehensive application development and management, including but not limited to dependency injection, aspect-oriented programming, and transaction management. Hibernate, with its efficient ORM capabilities, ensures smooth data transactions and interactions, thereby enhancing the application’s performance and scalability. Understanding their distinct roles and how they complement each other is crucial for developing robust, efficient Java applications.
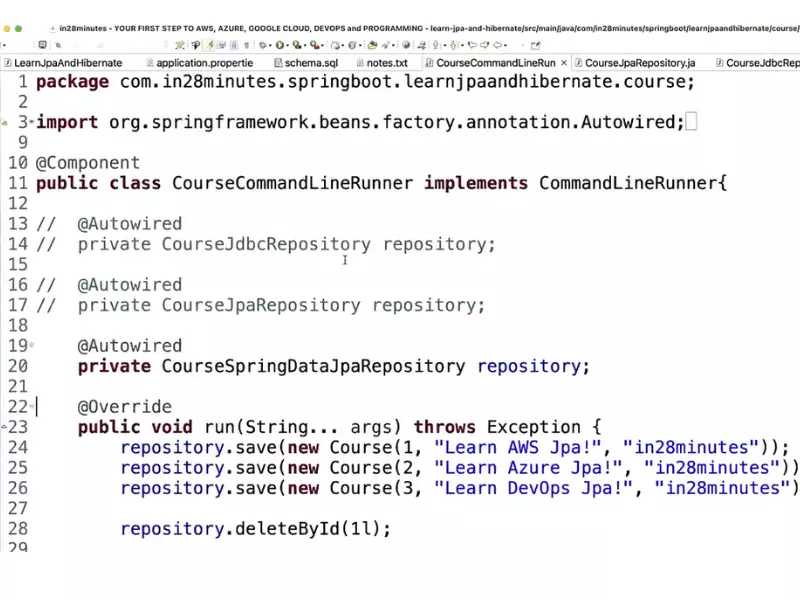
Spring Framework Basics
Core Features
The Spring Framework is a powerful, feature-rich framework for building Java applications. At its core, Spring simplifies the development process by providing comprehensive infrastructure support. This allows developers to focus more on their application’s business logic rather than the boilerplate code associated with setup and configuration. The core features of Spring include:
- Inversion of Control (IoC): This principle is the foundation of Spring, allowing for loose coupling through dependency injection. Instead of creating objects directly, Spring manages them and injects them as needed, making code easier to test and maintain.
- Aspect-Oriented Programming (AOP): AOP helps in separating cross-cutting concerns (like logging and security) from the business logic of the application. This results in a cleaner, more modular codebase.
- Transaction Management: Spring provides a consistent abstraction for transaction management that scales down to local transactions and scales up to global transactions (JTA).
- Spring MVC: A model-view-controller architecture that provides a powerful framework for building web applications. It is designed around a DispatcherServlet that dispatches requests to handlers, with configurable handler mappings, view resolution, locale, time zone, and theme resolution.
Spring Modules
Spring is modular, consisting of several modules that provide a wide range of functionality. These modules are grouped into Core Container, Data Access/Integration, Web, AOP (Aspect-Oriented Programming), Instrumentation, Messaging, and Test, among others. Important modules include:
- Spring Core Container: Includes the Spring Core, Beans, Context, and Expression Language modules, providing the fundamental parts of the framework.
- Spring Data Access/Integration: Covers JDBC, ORM, JMS, and Transactions modules for integrating with database and messaging systems.
- Spring Web: Encompasses Web, Web-MVC, Web-Socket, and Web-Portlet for creating web applications and services.
Hibernate Basics
Core Features
Hibernate is an Object-Relational Mapping (ORM) tool for Java, focusing on data persistence as its primary function. It simplifies the interaction between Java applications and databases by mapping Java classes to database tables and Java data types to SQL data types. Hibernate’s core features include:
- Object-Relational Mapping (ORM): Automates the mapping between Java objects and database tables to simplify data querying and manipulation.
- HQL (Hibernate Query Language): An object-oriented query language that simplifies data access and manipulation.
- Caching: Hibernate includes first-level cache by default and supports second-level cache and query cache, reducing the number of database queries, which significantly improves performance.
- Lazy Loading: Enhances performance by loading child objects on demand rather than at the time their parent is loaded.
- Automatic Dirty Checking: Automatically updates the database when an object’s state changes, without requiring explicit save or update calls.
Hibernate Architecture
The architecture of Hibernate is designed to act as a bridge between Java application objects and the database. Key components of Hibernate architecture include:
- SessionFactory: A thread-safe, immutable cache of compiled mappings for a single database. It provides Session instances to handle the actual database operations.
- Session: A single-threaded, short-lived object representing a conversation between the application and the database. It wraps a JDBC connection.
- Transaction: Manages a single unit of work concerning the database.
- ConnectionProvider: Manages the database connections.
- TransactionFactory: Handles transaction abstraction and management.
Configuration and Setup
Spring Setup
Setting up Spring in a project involves several steps, which can vary depending on the project’s specific needs and the build tool being used (like Maven or Gradle). Generally, the setup includes:
- Adding Spring Dependencies: Include Spring Boot starters or specific Spring module dependencies in your project’s build configuration file.
- Configuration: Use application.properties or application.yml for application-wide properties, and define beans in the Spring application context.
- Bootstrapping: Initialize your Spring application using the
@SpringBootApplication
annotation and running the main method.
Hibernate Setup
Configuring Hibernate typically involves:
- Adding Hibernate Dependencies: Include Hibernate Core and any required vendor-specific database dialects in your project’s build file.
- Configuring Hibernate: This can be done through a
hibernate.cfg.xml
file or programmatically in your application. You’ll need to specify the database connection properties, entity classes, and other settings. - SessionFactory Creation: Create a
SessionFactory
instance to manage sessions with the database.
Data Management
Spring Data Handling
Spring provides extensive support for data management through its Data Access/Integration layer, which includes:
- JdbcTemplate: Simplifies JDBC operations, catching exceptions and reducing boilerplate code.
- Repository Abstractions: Spring Data repositories abstract the data layer, making CRUD operations simpler and reducing boilerplate code.
Hibernate ORM
Hibernate’s ORM capabilities allow for efficient data management by mapping Java objects to database tables. This includes:
- CRUD Operations: Simplified by Hibernate’s session methods like
save()
,update()
, anddelete()
. - Querying: Supports HQL and criteria queries to retrieve data efficiently.
Transaction Management
Spring Approach
Spring’s transaction management offers a consistent programming model across different transaction APIs like JTA, JDBC, Hibernate, JPA, and others. It includes:
- Declarative Transaction Management: Using
@Transactional
annotation to define transactional behavior on methods. - Programmatic Transaction Management: Through the use of the
TransactionTemplate
for managing transaction boundaries programmatically.
Hibernate Approach
Hibernate manages transactions through the Session
and Transaction
interfaces. It allows for:
- Local Transactions: Managed through the Hibernate
Session
. - JTA Transactions: For global transactions, typically in a JEE environment, Hibernate integrates with JTA and container-managed transactions.
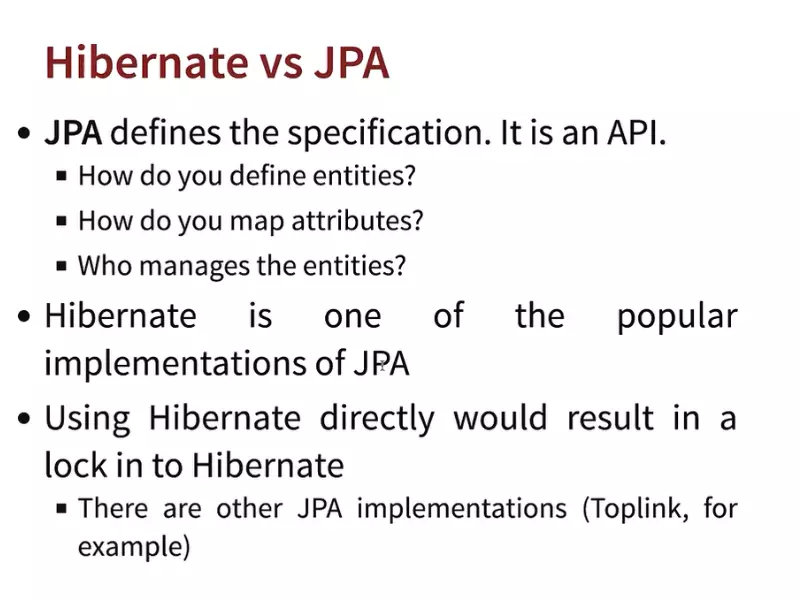
Dependency Injection
Spring DI Mechanism
Dependency Injection (DI) in Spring is a design pattern that allows a class’s dependencies to be injected at runtime rather than at compile time. This approach promotes loose coupling, making applications easier to test and maintain. Spring supports two types of DI:
- Constructor Injection: Dependencies are provided through a class’s constructor.
- Setter Injection: Dependencies are set through JavaBean properties.
Spring DI mechanism works by defining beans and their dependencies in a Spring configuration file or annotations in the class. The Spring container then injects these dependencies when it creates the beans. This process is fundamental to almost all Spring applications, enabling developers to manage complex applications with ease.
Comparison with Hibernate
While Hibernate does not offer a DI mechanism as advanced as Spring, it does manage object-relational mapping, which can be seen as a form of dependency management between objects and their data sources. However, Spring’s DI is more about managing class dependencies, while Hibernate focuses on data persistence and retrieval.
AOP Support
Aspect-Oriented Programming in Spring
Aspect-Oriented Programming (AOP) in Spring allows developers to define cross-cutting concerns that can be applied across different points of an application, such as logging, security, or transaction management. This is achieved without modifying the actual business logic, leading to a cleaner and more maintainable codebase. Key concepts in Spring AOP include:
- Aspects: Modules that crosscut multiple classes.
- Join Points: Points in the program execution where an aspect can be applied.
- Advice: Action taken by an aspect at a particular join point.
- Pointcuts: Expressions that select one or more join points.
Hibernate’s AOP Capabilities
While Hibernate is not primarily designed for AOP, it can work seamlessly with Spring AOP to handle concerns related to data access and transactions. For instance, Spring AOP can be used to declare transactional advice around methods that interact with Hibernate, ensuring consistent and efficient transaction management.
Integration Capabilities
Spring with Other Frameworks
Spring’s design philosophy makes it highly compatible with other frameworks, offering extensive integration capabilities. This allows Spring to serve as the foundation for complex applications that require functionalities beyond its scope. Key integration points include:
- Web Frameworks: Spring MVC can integrate with other web frameworks like Struts or JSF, allowing for flexible web application development.
- ORM Tools: Beyond Hibernate, Spring offers integration with other ORM tools like JPA, JDO, and iBatis, providing developers with a choice of data access strategies.
- Enterprise Services: Spring integrates with JMS, JNDI, Email, Scheduling, and other enterprise services, enabling rich application functionalities.
Hibernate Integration Points
Hibernate integrates well with various Java technologies and frameworks, enhancing its ORM capabilities. Notable integrations include:
- JPA: Hibernate can be used as the provider for the Java Persistence API, allowing for standard ORM functionality.
- Spring: As previously mentioned, Hibernate’s integration with Spring is robust, offering seamless transaction and dependency management.
Performance
Spring Performance Features
Spring offers various features and techniques to enhance application performance, including:
- Caching: Spring’s caching abstraction allows for easy caching integration, improving performance by reducing database load.
- Lazy Loading: Spring supports lazy initialization of beans, which can improve startup time and reduce memory footprint.
- Connection Pooling: Integration with connection pooling libraries ensures efficient database connectivity and resource utilization.
Hibernate Performance Optimization
Hibernate provides several strategies to optimize performance, such as:
- Lazy Loading: By default, Hibernate doesn’t load associated objects until they are accessed, reducing initial load times.
- Batch Processing: Hibernate allows for batch processing of SQL statements, reducing database round trips.
- Caching: Hibernate supports first-level and second-level caching, significantly reducing the number of database queries.
Use Cases
Ideal Scenarios for Spring
Spring is best suited for:
- Enterprise Applications: Where complex business logic needs to be encapsulated within a flexible, loosely coupled architecture.
- Web Applications: Spring MVC provides a powerful framework for building dynamic websites and RESTful web services.
- Microservices: Spring Boot makes it easy to create stand-alone, production-grade Spring-based applications that you can “just run”.
Ideal Scenarios for Hibernate
Hibernate excels in:
- ORM Requirements: Applications that require complex interactions with databases and benefit from ORM capabilities.
- Legacy Database Integration: Projects that need to work with existing database schemas without modifying them extensively.
Choosing Between Spring and Hibernate
Project Requirements
The choice between Spring and Hibernate depends on the project’s specific needs. If the project requires comprehensive application development capabilities including web development, security, and messaging, Spring is the go-to framework. For projects focused primarily on database interaction and ORM, Hibernate may be the better choice.
Developer Expertise
The decision also hinges on the development team’s expertise. Teams familiar with Spring’s ecosystem will likely find it easier to implement complex application architectures. Conversely, teams with a strong understanding of database design and ORM might lean towards Hibernate for its powerful data handling features.
FAQs
How Do Spring and Hibernate Work Together?
Spring and Hibernate can be integrated to leverage the strengths of both frameworks, offering a powerful combination for Java development. Spring’s comprehensive infrastructure support enhances application development and management, while Hibernate’s efficient ORM capabilities simplify database interactions. This integration facilitates streamlined data persistence and retrieval within a Spring-managed environment, enhancing application performance and scalability.
Can Spring Be Used Without Hibernate?
Yes, Spring can be used without Hibernate. Spring is a versatile framework that provides a wide range of functionality beyond data persistence, including web application development, security, messaging, and more. While Hibernate adds value in terms of ORM capabilities, Spring’s architecture allows for the use of alternative data access technologies or its own template-based approach to managing database interactions.
Is Hibernate Necessary for Java Development?
While Hibernate is not strictly necessary for Java development, it offers significant advantages for applications that require complex data handling and persistence. Its ORM capabilities streamline the mapping of Java objects to database tables, simplifying CRUD operations and reducing the amount of boilerplate code. However, developers can choose from other ORM tools or direct database interaction methods based on their project requirements.
What Are the Advantages of Using Spring Over Hibernate?
The advantages of using Spring over Hibernate lie in Spring’s broader scope and functionality. Spring provides a complete framework for all aspects of application development, from web applications to security and transaction management. It offers a more comprehensive solution for developers seeking an all-encompassing framework, while Hibernate focuses specifically on data persistence through ORM.
Conclusion
The distinction between Spring and Hibernate encapsulates a broader conversation about selecting the right tools for specific aspects of Java development. While Spring offers a wide-ranging framework designed to cater to various facets of application development, Hibernate specializes in streamlining database interactions through its ORM capabilities. Understanding the unique advantages and applications of each framework allows developers to make informed decisions, ensuring the development of efficient, scalable, and robust Java applications.
Choosing between Spring and Hibernate, or more appropriately, understanding how to leverage them in conjunction, is crucial for maximizing application performance and developer productivity. This comprehension enables the development of applications that are not only technically sound but also aligned with the project’s requirements and goals. The synergy between Spring and Hibernate, when harnessed correctly, paves the way for creating advanced Java applications that stand the test of time.