In the digital world, the creation and execution of software hinge on two critical components: source code and bytecode. These elements serve as the backbone of software development, each playing a unique role in transforming ideas into functional applications. Source code acts as the blueprint, written in languages that are human-readable, such as Python, Java, or C++. Bytecode, on the other hand, serves as an intermediary form, a bridge between human ideas and machine execution.
Source code is written by developers in various programming languages and is understandable by humans. It needs to be compiled or interpreted to run on a computer. Bytecode is the result of compiling source code, an optimized, intermediate representation that can be executed by a virtual machine. Unlike source code, bytecode is not directly readable by humans but can be executed across different platforms without modification, offering a level of abstraction and portability not found in raw source code.
The distinction between source code and bytecode is pivotal for understanding how software operates across diverse environments. Source code provides the instructions in a human-readable format, which is then transformed into bytecode, an efficient, machine-interpretable format. This process enhances software portability and efficiency, allowing applications to run on any platform with a compatible virtual machine. The relationship between these two components underscores the intricate balance between human creativity and machine precision in the realm of software development.
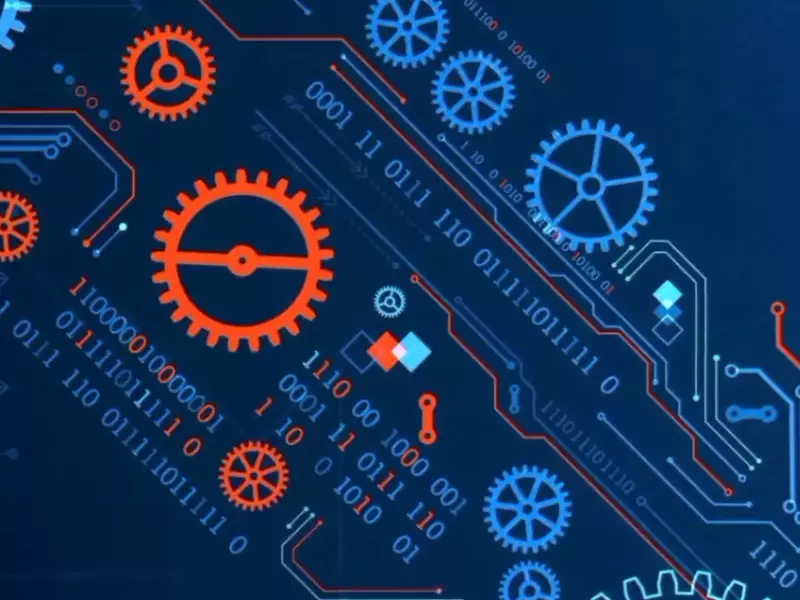
Source Code Basics
Nature of Source Code
At its core, source code is the set of instructions written by developers to be executed by a computer. It’s crafted in a human-readable format, meaning that the code is written in a way that humans can understand and modify. This format allows developers to outline the functionality and behavior of software applications.
Languages Involved
Programming languages are the tools developers use to write source code. These languages can vary widely in syntax and use, but all serve the purpose of translating human logic into instructions a computer can execute. Common examples include Python, Java, C++, and JavaScript. Each language has its own set of rules (syntax) and is designed with specific goals in mind, catering to different types of projects and performance needs.
Creation Process
Writing and Editing
The process of creating source code involves several steps, primarily focused on writing and editing. Developers write code to define how the software should operate, solve problems, and perform tasks. This process often involves:
- Brainstorming solutions to problems
- Writing the initial code
- Testing the code to find and fix errors
- Refining and optimizing the code for better performance
Tools Used for Development
To aid in the development process, several tools are employed:
- Integrated Development Environments (IDEs) like Visual Studio Code or IntelliJ IDEA, which provide a comprehensive environment for coding, with built-in tools for editing, debugging, and running code.
- Text editors such as Sublime Text or Atom, for those who prefer a simpler, more lightweight coding environment.
- Version control systems like Git, which help in tracking changes to the codebase and facilitating collaboration among developers.
Bytecode Explained
Nature of Bytecode
Bytecode is an intermediate form of code, generated from compiling source code. It is not directly readable by humans but can be understood by virtual machines (VMs) or interpreters. Bytecode occupies a middle ground between human-readable source code and the binary machine code that computers execute directly.
Intermediate Form
Being an intermediate form, bytecode acts as a bridge, enabling code to be written once and then run on multiple platforms without modification. This is because the virtual machine that executes the bytecode is designed to operate on various operating systems and hardware configurations.
Comparison with Machine Code
Unlike machine code, which is the lowest-level representation of code specific to processor architecture, bytecode is higher-level and more abstract. Machine code is designed to be executed directly by the CPU, making it incredibly fast but also platform-specific. Bytecode, meanwhile, needs a virtual machine to interpret or compile it into machine code at runtime, providing portability at the cost of some performance.
Generation Process
Compilation of Source Code
The transformation of source code into bytecode is achieved through compilation. This process involves reading the human-readable instructions and converting them into an optimized, machine-interpretable format. The compiler, a key tool in this process, analyzes the source code, optimizes it for performance, and then generates bytecode.
Role of Compilers and Interpreters
Compilers and interpreters serve slightly different roles in the execution of code:
- Compilers translate source code into bytecode or machine code before the program is run. This results in faster execution time since the code is already in a directly executable form.
- Interpreters directly execute source code instructions without compiling them into bytecode first, which can be slower but allows for more flexibility in script execution and debugging.
Key Differences
Readability
The main difference in readability between source code and bytecode is their intended audience:
- Source code is written for humans. It’s structured, documented, and meant to be read and understood by developers.
- Bytecode, on the other hand, is for machines, specifically virtual machines. It’s a set of optimized instructions that are not meant to be directly interpreted by humans.
Execution
When it comes to execution, the distinction becomes clearer:
- Source code must be interpreted or compiled to be executed, either turning into bytecode or directly into machine code.
- Bytecode runs inside a virtual machine (VM) which interprets it or compiles it just-in-time (JIT) into machine code for execution. This adds a layer of abstraction, allowing bytecode to be platform-independent.
Portability
Portability is a significant advantage of bytecode over source code:
- Source code is often tied to a specific language’s runtime or a platform’s characteristics. While it can be shared across platforms, it might require modifications or different runtime environments.
- Bytecode is designed to be cross-platform, able to run on any device with a compatible virtual machine, enhancing software distribution and flexibility.
Performance
The performance of software can be affected by whether it’s executed from source code or bytecode:
- Compilation time and execution speed vary, with source code needing to be compiled, which can be time-consuming, but potentially resulting in faster execution as machine code.
- Bytecode is generally faster to start executing since it’s already compiled, but it may run slower than native machine code due to the overhead of the virtual machine.
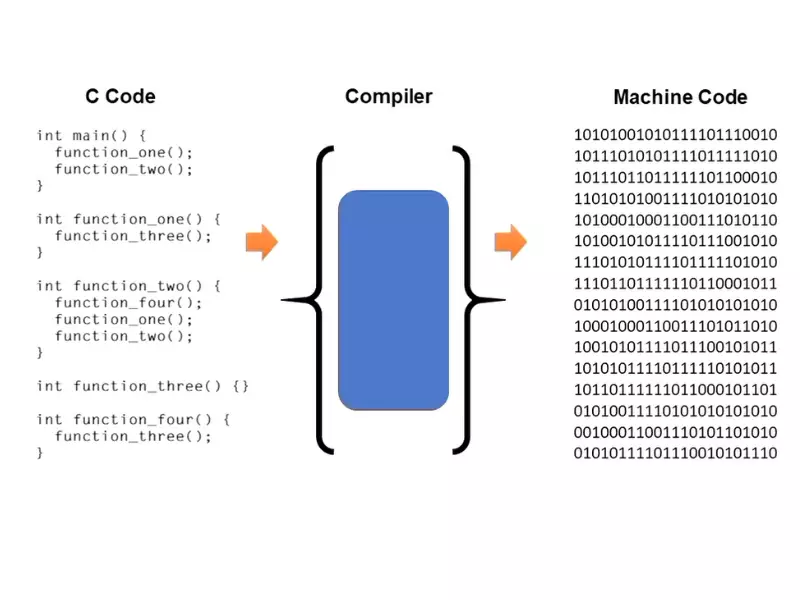
Practical Implications
Application Development
Choice of Programming Languages
The selection of a programming language is a crucial decision in application development, significantly impacting the project’s speed and flexibility. Languages like Python are praised for their simplicity and rapid development capabilities, making them ideal for startups and fast-paced environments. On the other hand, languages such as C++ offer extensive control over system resources, preferred in applications where performance is critical. Choosing the right language involves balancing between development speed, application performance, and the project’s specific needs.
- Python and JavaScript enhance development speed due to their simplicity and extensive libraries.
- Java and C# provide a balance between speed and performance, with robust frameworks and virtual machines that simplify cross-platform development.
- C++ is chosen for system-level software where control and efficiency are paramount.
Impact on Development Speed and Flexibility
Programming languages and their associated tools significantly affect development speed and flexibility. High-level languages with extensive standard libraries and frameworks allow developers to implement complex features quickly, while lower-level languages may slow development but offer greater control and efficiency.
- High-level languages: Rapid prototyping and iteration
- Low-level languages: More time-consuming but optimized performance
Software Distribution
Security Aspects
Security is a paramount concern in software distribution, impacting how software is packaged, distributed, and updated. The transition from source code to bytecode can introduce security measures that protect the code from tampering and unauthorized access. Techniques such as code obfuscation and encryption make bytecode more secure but can also complicate debugging and maintenance.
- Code signing ensures the authenticity of the software, verifying that it hasn’t been tampered with since its distribution.
- Secure distribution channels protect the integrity of software updates and downloads.
Distribution Formats
Software can be distributed in various formats, each with its own set of advantages. Executable binaries are platform-specific and offer the fastest execution but the least flexibility. Bytecode formats, such as Java’s JAR files, provide a balance between performance and portability, allowing the same package to run across different platforms with a compatible VM.
- Source code distribution allows maximum transparency and customization but requires the end-user to compile the software, which can be a barrier for non-developers.
- Containerization (e.g., Docker containers) packages the software and its environment, ensuring consistency across development, testing, and production environments.
Execution Environments
Virtual Machines and Their Role
Virtual machines (VMs) play a crucial role in modern software development and execution by providing an abstraction layer between the bytecode and the physical hardware. This allows applications written in bytecode to run on any platform with a compatible VM, enhancing portability and simplifying development.
- JVM (Java Virtual Machine): Executes Java bytecode, enabling Java applications to run on any device that has the JVM installed, regardless of the underlying hardware and operating system.
- .NET CLR (Common Language Runtime): Executes applications written in .NET languages, providing services such as memory management, security, and exception handling.
Examples: JVM, .NET CLR
- JVM is renowned for its “write once, run anywhere” philosophy, attributed to Java’s platform-independent bytecode. It has powered countless enterprise applications, Android apps, and large-scale systems.
- .NET CLR supports multiple programming languages, including C#, F#, and VB.NET, allowing developers to choose the language best suited for their application while still benefiting from the CLR’s execution and security features.
Both environments offer extensive libraries and frameworks that streamline the development of web, mobile, and desktop applications, ensuring that applications are secure, efficient, and maintainable.
The Future of Execution Environments
The evolution of execution environments like JVM and .NET CLR continues to shape the future of software development and distribution. Advances in cloud computing, containerization, and microservices architecture are making applications more scalable, resilient, and easier to deploy. These technologies leverage the strengths of virtual machines and bytecode to deliver software that meets the demands of modern businesses and consumers.
- Cloud-based VMs offer on-demand scalability and global distribution, reducing the overhead of managing physical servers.
- Microservices can be deployed independently in containers, each running in its own VM, enhancing the agility and reliability of complex applications.
Frequently Asked Questions
What is source code?
Source code refers to the set of instructions written by developers in a programming language that is understandable by humans. It forms the original codebase of a software application, outlining the functionality and logic that the software is intended to perform.
How is bytecode generated from source code?
Bytecode is generated from source code through a process called compilation. This process involves translating the high-level, human-readable instructions of the source code into an optimized, intermediate form that can be understood and executed by a virtual machine, making the software platform-independent.
Why is bytecode considered platform-independent?
Bytecode is considered platform-independent because it can be executed on any device that has a compatible virtual machine (VM). The VM interprets the bytecode for the device’s specific hardware, allowing the same bytecode to run on different operating systems and hardware configurations without needing to be rewritten or recompiled.
Can bytecode be converted back to source code?
While bytecode can technically be decompiled to a form that resembles source code, the resulting code may not be identical to the original source code. Decompilation processes can recover the structure and flow of the program but often lose comments, variable names, and certain structures, making the recovered code less readable and potentially harder to understand.
Conclusion
The nuanced distinction between source code and bytecode plays a crucial role in the development, execution, and distribution of software across various platforms. Source code, with its human-readable syntax, lays the groundwork for software creation, embodying the logic and functionality envisioned by its developers. Bytecode, as an intermediary form, bridges the gap between human creativity and machine execution, ensuring that applications can run efficiently and portably across different environments.
Understanding the interplay between source code and bytecode not only demystifies the process of software development but also highlights the technological advancements that allow applications to transcend the boundaries of hardware and operating systems. This knowledge equips developers, learners, and enthusiasts with a deeper appreciation of the complexities and elegance behind the software that powers our digital world.