Sorting algorithms are fundamental to the field of computer science, enabling the organization of data in a specific order to improve efficiency and manageability. These algorithms vary widely in terms of methodology, complexity, and use case scenarios, with each possessing unique strengths and weaknesses. Among the plethora of sorting methods, Insertion Sort and Selection Sort stand out for their simplicity and frequent use in introductory computer science courses.
The difference between Insertion Sort and Selection Sort lies primarily in their approach to arranging data. Insertion Sort builds the sorted array one element at a time, inserting each new element into its correct position within the already sorted part. On the other hand, Selection Sort systematically searches for the smallest (or largest) element from the unsorted portion and places it at the end of the sorted section until all elements are properly ordered.
Both algorithms play a crucial role in understanding how data can be efficiently sorted and manipulated. While Insertion Sort is celebrated for its adaptiveness and efficiency in handling small or partially sorted datasets, Selection Sort is appreciated for its simplicity and straightforward implementation, making it an excellent educational tool for those new to algorithm design. Despite their differences, both sorting techniques provide a foundation for tackling more complex sorting challenges and understanding algorithmic efficiency.
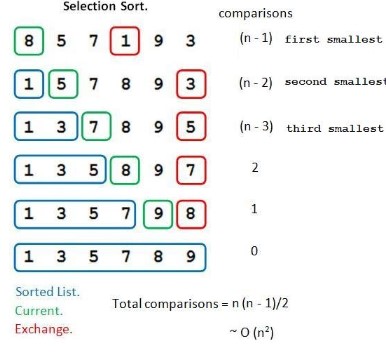
Sorting Basics
Concept of Sorting
Definition and Purpose
Sorting is the process of arranging data in a particular order, most commonly in ascending or descending sequence. The primary purpose of sorting is to increase the efficiency of other operations, such as searching and merging, by organizing data in a manner that is predictable and easily navigable. For developers and algorithms, sorting is a fundamental task that underpins the performance and usability of applications across a variety of domains.
Sorting Algorithms Overview
Sorting algorithms are methods designed to order the elements of a list according to a specific rule. There are several sorting algorithms, each with its unique mechanics and use cases. Common examples include:
- Bubble Sort: Simple but inefficient for large datasets, it repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- Quick Sort: Highly efficient for large datasets, it divides the list into two parts based on a pivot element and then recursively sorts the sub-lists.
- Merge Sort: Also efficient and useful for large datasets, it divides the list into the smallest unit (1 element), then merges them back together in order, creating a sorted list.
Insertion Sort
How It Works
Insertion Sort is a simple sorting algorithm that builds the final sorted array one item at a time. Here’s a step-by-step process:
- Start with the second element of the array.
- Compare this element with the ones before it, moving each one step to the right until finding the correct position for the new element.
- Insert the new element into its correct position.
- Repeat the process for all elements in the array.
Key Features
Adaptiveness
Insertion Sort is adaptive, meaning it works well with partially sorted arrays, reducing the number of comparisons and swaps needed.
Stability
It is stable; it does not change the relative order of elements with equal keys. This property is crucial for sorting complex structures where secondary sorting is important.
Use Cases
- Small Datasets: Highly efficient for small to medium-sized datasets due to its simplicity.
- Nearly Sorted Datasets: Exceptionally efficient because it minimizes the number of necessary operations.
- Online Sorting: Suitable for scenarios where data is continuously added and needs to be sorted.
Selection Sort
How It Works
Selection Sort systematically selects the minimum (or maximum) from the list and swaps it with the element in the current position. Here is the step-by-step explanation:
- Identify the smallest (or largest) element in the array.
- Swap it with the element in the current position.
- Move the current position one step right, and repeat the process for the remaining unsorted elements.
Key Features
Simplicity
The primary advantage of Selection Sort is its simplicity, making it easy to understand and implement.
Lack of Adaptiveness
Unlike Insertion Sort, Selection Sort is not adaptive; it has a fixed number of comparisons and does not benefit from an initially sorted array.
Use Cases
- Educational Purposes: Due to its simplicity, it’s often used to teach sorting algorithm concepts.
- Small Datasets: While not as efficient as some other algorithms for larger datasets, it can perform adequately for small datasets.
Comparative Analysis
Performance Comparison
Time Complexity
When comparing Insertion Sort and Selection Sort, understanding their time complexity is essential. Both algorithms have an average and worst-case time complexity of O(n^2), where n is the number of items in the array. This means that for both sorting methods, the time it takes to sort the elements increases quadratically as the number of elements increases. However, Insertion Sort tends to perform slightly better in practice due to its adaptiveness, especially on nearly sorted or small datasets.
Space Complexity
Regarding space complexity, both Insertion Sort and Selection Sort excel as in-place sorting algorithms, requiring no additional storage beyond what is needed for the original array. The space complexity for both is O(1), indicating that the space required to sort the data does not increase with the size of the dataset.
Adaptiveness and Stability
Definitions
- Adaptiveness refers to an algorithm’s ability to perform better given a partially sorted list. An adaptive algorithm will complete its task quicker if the elements are already somewhat in order.
- Stability means that if two elements have the same key, their relative order will be the same in the input and the output.
Comparative Discussion
Insertion Sort is adaptive, which means it can sort a partially sorted array much faster than a completely unsorted one. This adaptiveness significantly enhances its efficiency in practical scenarios where data may already be partially organized. On the stability front, Insertion Sort is stable, making it suitable for sorting data where the relative order of duplicate values needs to be preserved.
In contrast, Selection Sort lacks adaptiveness; it will perform the same number of operations regardless of the initial order of the data. This lack of adaptiveness can lead to unnecessary comparisons and swaps, making it less efficient than Insertion Sort for nearly sorted data. Selection Sort is also stable, but this stability comes with the trade-off of its non-adaptive nature.
Practical Applications
Best Use Scenarios for Each
- Insertion Sort is best used in scenarios involving small datasets, partially sorted datasets, or when stability is a priority. Its adaptiveness makes it highly efficient for these cases, especially for online sorting applications where data is continuously added and needs sorting.
- Selection Sort, with its simplicity, is ideally suited for educational purposes and scenarios where the simplicity of the code is more critical than performance. Its non-adaptive nature and predictable operation make it a useful tool for demonstrating sorting algorithm concepts, despite its inefficiencies with larger datasets.
Pros and Cons
Insertion Sort Advantages
- Efficiency on small datasets: Excels at sorting small arrays.
- Adaptiveness: Performs exceptionally well on nearly sorted datasets.
- Stability: Maintains the relative order of duplicate elements, which is crucial for complex data sorting.
Insertion Sort Disadvantages
- Limitations with large datasets: Its performance significantly drops as the dataset size increases due to its O(n^2) time complexity.
Selection Sort Advantages
- Simplicity: Easy to understand and implement, making it an excellent educational tool.
- Predictable performance: Performs consistently regardless of the dataset’s initial order.
Selection Sort Disadvantages
- Inefficiency on large datasets: Due to its O(n^2) time complexity, it becomes impractical for larger datasets.
- Lack of adaptiveness: Does not take advantage of the dataset’s initial order, potentially leading to unnecessary operations.
Choosing Between Insertion and Selection Sort
When deciding between Insertion Sort and Selection Sort, several factors come into play, determining which algorithm is more suited to a particular application.
Factors to Consider
- Data size: For small datasets, both algorithms can be efficient, but as the size increases, the inefficiencies of O(n^2) algorithms become more pronounced.
- Data structure: If the data is already partially sorted or expected to be added incrementally, Insertion Sort’s adaptiveness offers a clear advantage.
- Application requirements: The need for stability, simplicity, or educational value can also guide the choice. Insertion Sort’s stability makes it preferable for sorting complex data structures, while Selection Sort’s simplicity and predictability can be advantageous in educational settings or where simplicity is valued over raw performance.
Frequently Asked Questions
What is Sorting in Computer Science?
Sorting in computer science refers to the process of arranging data elements in a specified order, either ascending or descending, to improve data organization and access efficiency. This process is pivotal in optimizing searches, data management tasks, and algorithm performance, making it a fundamental concept in the field.
Why is Algorithm Complexity Important?
Algorithm complexity is crucial because it determines the efficiency of an algorithm in terms of time and space. Understanding complexity helps developers predict how algorithms will scale with increasing data volumes, guiding the selection of the most appropriate algorithm for a given task and ensuring optimal performance and resource utilization.
How do Insertion Sort and Selection Sort Differ in Performance?
Insertion Sort and Selection Sort differ in performance primarily due to their time complexity. Insertion Sort is generally faster for small or partially sorted datasets due to its adaptive nature, while Selection Sort maintains a consistent performance regardless of the initial order of elements, making it less efficient for larger datasets.
Can Insertion Sort and Selection Sort be Used Interchangeably?
While Insertion Sort and Selection Sort can be used to achieve the same outcome—sorted data—their efficiency and suitability vary depending on the dataset size, initial order, and specific application requirements. Choosing between them depends on the context, with Insertion Sort favored for smaller or nearly sorted datasets and Selection Sort for simplicity and teaching purposes.
Conclusion
In conclusion, Insertion Sort and Selection Sort are both pivotal in the landscape of sorting algorithms, each with its methodology and area of application. Their differences highlight the diversity of approaches in algorithm design, offering valuable insights into efficiency, adaptiveness, and simplicity. As we navigate through the complexities of data sorting, understanding these algorithms provides a solid foundation for exploring more advanced sorting techniques and their applications in real-world scenarios.
Furthermore, the choice between Insertion Sort and Selection Sort underscores the importance of context in algorithm selection. It reflects a broader principle in computer science: that the best solution often depends on the specific problem at hand, requiring a nuanced understanding of algorithm characteristics and performance metrics. By appreciating these sorting algorithms, we gain not only practical tools for data organization but also deeper insights into the principles guiding efficient algorithm design.