In the realm of software development, two critical components play pivotal roles in the construction and execution of applications: header files and library files. These elements are fundamental to the efficient development of software, serving as the building blocks that developers rely on to integrate pre-existing code into their projects. Their correct use and understanding are essential for any developer aiming to create robust and maintainable software.
Header files are primarily used for declarations, acting as a bridge that connects different parts of a program by declaring functions, variables, and classes to be used across various source files. On the other hand, library files contain the actual implementation of these declarations—compiled code that can be linked to your program, providing functionality without needing to write the code from scratch. This separation between declaration and implementation is what allows developers to write more manageable and modular code.
Understanding the distinction between these two types of files is crucial for effective software development. Header files (.h, .hpp) facilitate the sharing of interfaces, enabling code modularity and reuse, while library files (.lib, .a, .dll, .so) encapsulate implementation, allowing for code functionality to be easily incorporated into different projects. This clear separation supports the development of scalable, maintainable, and efficient software systems.
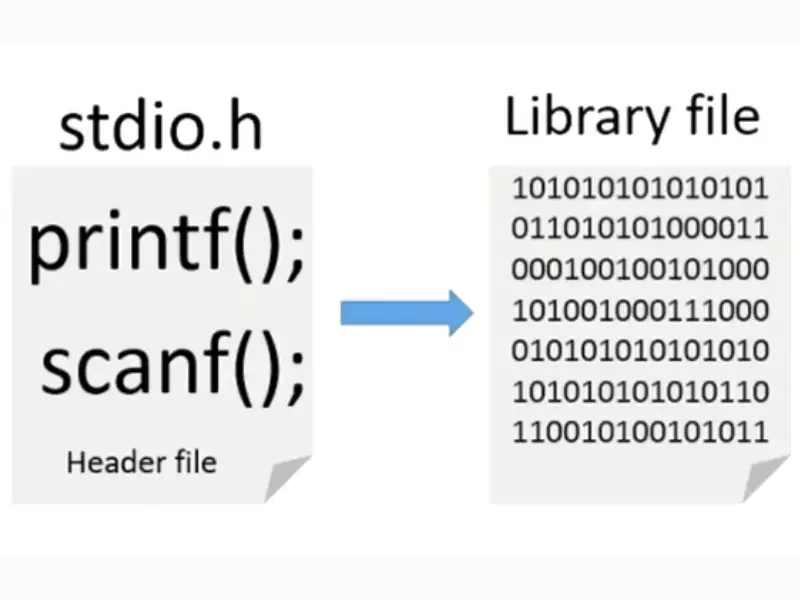
Core Concepts
Header Files Explained
In the world of C and C++ programming, header files play a critical role. These files, typically with extensions .h
or .hpp
, serve as the foundation for sharing function declarations, macro definitions, and other important declarations across multiple source files. The primary purpose of header files is to declare the interface to a set of functions or classes without defining their actual implementation. This enables developers to separate the implementation details of their code from its interface, fostering modularity and reusability.
- Function Declarations: Allows the compiler to know about the existence of a function that will be defined elsewhere.
- Macro Definitions: Used to define constants or macros that can be used across multiple files.
- Type Definitions: Declare new data types and structures for use throughout the program.
By including the same header file in various source files, programmers can ensure that their code is consistent, reliable, and easier to navigate. This practice significantly enhances the maintainability of large software projects, making header files indispensable in the software development lifecycle.
Library Files Overview
Library files stand at the heart of efficient software development, offering a repository of precompiled code that developers can include in their applications. These files come in two main flavors: static libraries (.lib, .a) and dynamic libraries (.dll, .so), each serving a distinct purpose in the development process.
- Static Libraries: These are collections of object files that are linked into the application at compile time. The code contained within a static library becomes part of the executable, leading to larger binary sizes but offering the advantage of not requiring the library at runtime.
- Dynamic Libraries: Unlike static libraries, dynamic libraries are linked during the application’s runtime rather than at compile time. This means the application can share and use a single copy of the library code, which can reduce the overall size of the application and allow for updates without recompiling.
Library files are essential for code reusability, allowing developers to leverage a vast ecosystem of prewritten functionality. This not only speeds up the development process but also helps ensure that the code is more reliable and tested, as it relies on well-established libraries.
Key Differences
Purpose and Function
The distinction between header and library files primarily lies in their purpose and function. Header files are designed for declarations, serving as a blueprint for the elements (functions, variables, classes) that will be used in a program. Library files, on the other hand, contain the implementation of these declarations—the actual code that executes the tasks defined by the interface in the header files. This separation is crucial for maintaining clear boundaries between the structure of a program and its implementation details.
Compilation Process
Understanding the compilation process is vital for grasping how header and library files are utilized. During compilation, the compiler uses header files to check for syntax and to understand the structure of the program. The linker then uses library files to bind these declarations to their respective implementations. This process ensures that the final executable knows where to find the code it needs to run correctly.
- Header files are included at compile time, ensuring the compiler knows about the declarations.
- Library files are linked after the compilation, either statically (at link time) or dynamically (at runtime).
File Types
Different file types are associated with header and library files, each signifying the file’s role in the development process:
- Header Files:
.h
for C headers and.hpp
or.hxx
for C++ headers, indicating interface declarations. - Static Library Files:
.lib
in Windows or.a
in Unix/Linux, representing a collection of compiled code that can be statically linked. - Dynamic Library Files:
.dll
in Windows or.so
in Unix/Linux, used for sharing code at runtime.
These extensions help developers and the build system to quickly identify the nature of each file and how it should be used in the project.
Code Reusability
Both header and library files contribute significantly to code reusability and modular programming. By separating declarations from implementations, developers can easily share and reuse code across multiple projects. This not only speeds up development time but also helps in maintaining a consistent codebase.
- Header files allow for the easy inclusion of interfaces in different parts of a project.
- Library files enable the reuse of precompiled code, reducing duplication and errors.
Dependency Management
Dependency management becomes more streamlined with the use of header and library files. However, changes to these files can have widespread implications for a software project:
- Changes to a header file can require recompilation of all source files that include it, potentially introducing compatibility issues.
- Updates to a library file, especially dynamic libraries, can affect the runtime behavior of applications that depend on them, necessitating careful version control and distribution strategies.
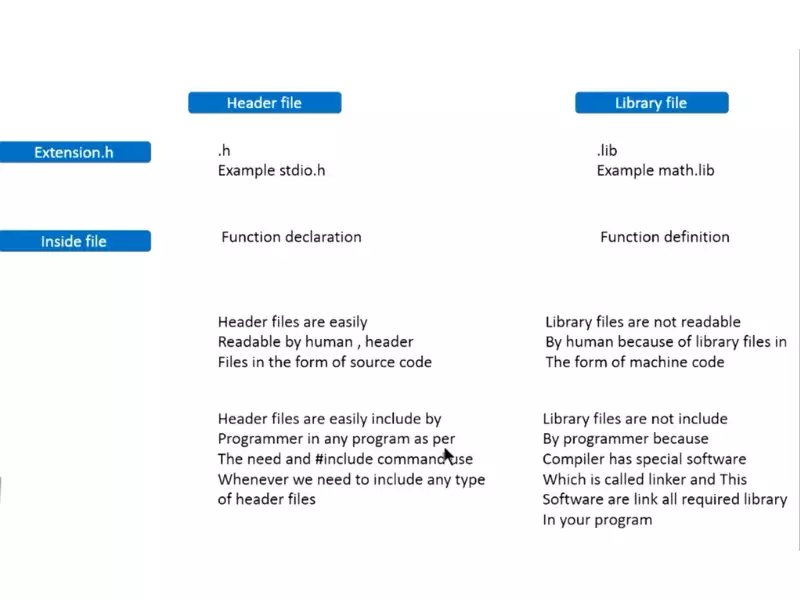
Practical Applications
Including Header Files
Correctly including header files in your source code is fundamental to ensuring that your programs compile and run as expected. Here’s how to do it right:
- Use Quotes for Local Headers: When including header files that you have created for your project, use double quotes. For example,
#include "myHeader.h"
tells the compiler to look for the header file in the same directory as the source file or in the specified search path. - Use Angle Brackets for System Headers: For standard library headers or those provided by third-party libraries, use angle brackets. For instance,
#include <iostream>
directs the compiler to search in standard system directories. - Guard Against Multiple Inclusions: To prevent the same header file from being included more than once, which can lead to errors, use include guards. This is typically done by defining a unique macro at the beginning of the header file and checking if it’s already defined:cppCopy code
#ifndef MY_HEADER_H #define MY_HEADER_H // Header file content #endif
Linking Library Files
Linking library files correctly is crucial for the proper execution of your software. Here’s a guide to linking both static and dynamic libraries:
- Static Libraries:
- When using a static library, the library’s contents are copied into the final executable during the linking phase. This increases the size of the executable but simplifies distribution.
- To link a static library, you might use a compiler flag like
-l
followed by the library name without the ‘lib’ prefix or the file extension. For example, if your static library file is namedlibmath.a
, you would use-lmath
in your compiler’s command line.
- Dynamic Libraries:
- Dynamic libraries are not included in the final executable. Instead, they are loaded at runtime. This keeps the executable size smaller and allows multiple programs to share the library’s code.
- Linking to a dynamic library involves specifying the library path during compilation using flags such as
-L
to specify the library directory and-l
for the library name. At runtime, ensure the library is in a location where your operating system can find it, such as by setting theLD_LIBRARY_PATH
environment variable on Linux.
Best Practices
Managing header and library files in large projects requires a strategic approach to maintain organizational clarity and code quality:
- Directory Structure: Organize your header and library files into clear directories. For instance, keep all your header files in an
include
directory and library files in alib
directory. This makes it easier to manage large codebases and simplifies the include and link paths for the compiler. - Naming Conventions: Adopt consistent naming conventions for your files. This helps in quickly identifying the purpose and scope of each file. For example, use prefixes or suffixes that indicate whether a file is a class, interface, or utility.
- Modular Design: Design your code in a modular fashion, with each header and corresponding library file encapsulating a coherent set of functionalities. This enhances reusability and maintainability.
Advanced Considerations
Cross-Platform Compatibility
Developing software that runs across different operating systems can present challenges with header and library files:
- Path Differences: Be aware of the differences in file paths and separators. Use conditional compilation or build-time variables to accommodate different platforms.
- Compatibility Issues: Test your code on all target platforms to ensure compatibility. This includes making sure that any third-party libraries you use are available and functional on those platforms.
- Build Tools: Utilize cross-platform build tools like CMake to abstract away many of the platform-specific details of managing header and library files.
Version Control
Integrating version control practices for your header and library files is essential for maintaining a stable and manageable code base:
- Track Changes: Use a version control system to track changes to your header and library files. This allows you to revert to previous versions if a new change introduces bugs or compatibility issues.
- Branching Strategies: Adopt branching strategies that allow for parallel development of features while keeping the main branch stable. This is particularly important for libraries used by multiple projects.
- Submodules and Packages: Consider using submodules (in Git) or package managers (like Conan for C++) for managing external libraries. This helps in keeping external dependencies organized and ensures that everyone is using the correct version.
Frequently Asked Questions
What is a header file?
A header file in programming languages like C and C++ is a file containing C declarations and macro definitions to be shared between several source files. It typically includes function prototypes, definitions of types and variables, and #include directives for other header files, facilitating code reusability and modularity by allowing the same header file to be included in multiple source files.
How do library files work?
Library files contain compiled code that can be linked to a program at compile-time (static libraries) or at run-time (dynamic libraries). They provide pre-written functionality that can be reused across multiple programs, reducing development time by eliminating the need to write frequently used code from scratch. Linking to library files enables the use of functions and classes without directly including their source code in a project.
Why separate declaration and implementation?
Separating declaration and implementation into header and library files, respectively, enhances code readability and reusability. It allows developers to interface with the code without knowing its underlying implementation details, facilitating easier maintenance and updates. This separation also aids in reducing compile times and managing dependencies more efficiently.
Can header files contain implementations?
While header files are primarily intended for declarations, they can contain implementations of templates, inline functions, and constexpr variables in C++. This practice, however, should be used judiciously to maintain clear separation between interface and implementation and to avoid potential issues with code bloat and multiple definition errors.
Conclusion
The distinction between header and library files is a cornerstone of modern software development, enabling developers to construct complex applications more efficiently. Header files provide the necessary declarations, making it possible to write code that is both modular and reusable, while library files offer the convenience of using precompiled code, thereby saving time and effort on implementation. This separation not only facilitates a cleaner and more organized codebase but also enhances the scalability and maintainability of software projects.
Recognizing and understanding the roles and differences of header and library files is crucial for any developer looking to advance their programming skills. It is this foundational knowledge that underpins efficient software development, allowing for the creation of robust applications that stand the test of time. Through the judicious use of these files, developers can harness the power of code reuse and modularity, paving the way for more innovative and complex software solutions.