Variables in programming serve as the cornerstone for storing, modifying, and retrieving data, enabling software to execute tasks dynamically and interactively. Among these, class and instance variables stand out for their distinct roles and behaviors within object-oriented programming. Their understanding is crucial for developers to efficiently manage data across different scopes and lifecycles of software applications.
Class variables are shared across all instances of a class, making them ideal for storing data that is consistent among each instance of that class. On the other hand, instance variables are unique to each instance, allowing for the individual characteristics of an instance to be stored and manipulated. This distinction plays a pivotal role in the design and functionality of software, impacting memory management, data integrity, and the behavior of applications.
In the realm of software development, class and instance variables are utilized to encapsulate and manage the state and behaviors of objects, respectively. Class variables, being static, provide a shared space that reflects values pertinent to the class as a whole. Conversely, instance variables offer a personalized space for each object, ensuring that individual attributes and states are maintained independently. This dichotomy not only enhances the modularity and reusability of code but also aids in crafting more organized and maintainable software architectures.
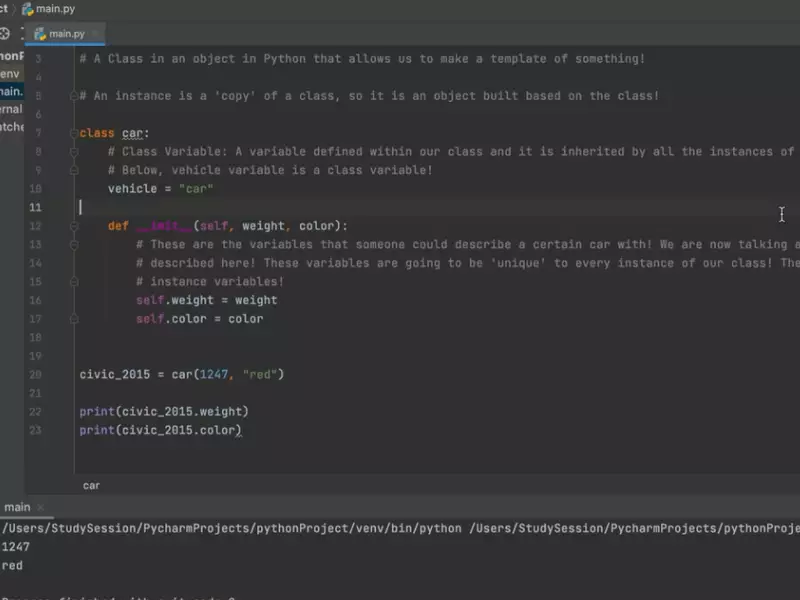
Difference Between Class and Instance Variables
Class Variables Explained
Definition and Characteristics
Class variables, also known as static variables, are defined within a class and outside any method. They are not tied to any particular instance of a class. Instead, class variables are shared across all instances of that class. This means that if the value of a class variable is changed in one instance, the change is reflected across all instances.
Class variables are typically used for constants or for fields that should be common to all instances. They are ideal for storing default values or shared data that is consistent across objects.
Common Uses
- Counting instances: Class variables can be used to keep track of the number of instances of a class that have been created.
- Configuration options: Shared configuration settings applicable to all objects.
- Shared resources: Such as a database connection pool.
Advantages and Limitations
Advantages:
- Memory efficiency: Since class variables are shared, they do not need to be replicated for each instance, saving memory.
- Data consistency: Ensures that all instances use a consistent set of data.
Limitations:
- Mutability: If mutable objects are used as class variables, they can be accidentally modified across all instances, leading to potential data integrity issues.
- Overuse: Overreliance on class variables can make the code harder to understand and maintain, especially in large projects.
Scope and Accessibility
Class variables are accessible from both instance methods and class methods. They are shared among instances, meaning they have a global scope within the class context.
Modification Impact
Modifying a class variable affects all instances of the class. This static nature can be useful but requires careful management to avoid unintended side effects.
Instance Variables Explained
Definition and Characteristics
Instance variables are tied to specific instances of a class. Each instance has its own copy of an instance variable, meaning changes made to an instance variable in one object do not affect the instance variables in other objects.
These variables are ideal for storing instance-specific data, allowing objects to maintain state independently of each other.
Personalization of Instances
Instance variables enable each object to have its unique state. This is crucial for object-oriented programming, allowing for the creation of diverse objects with the same class but different attributes.
Scope and Accessibility
Instance variables are only accessible within the context of their instance. This means that each object maintains its own set of data, leading to:
- Data encapsulation: Encouraging a modular and cohesive design.
- Data isolation: Ensuring that the state of an object cannot be inadvertently affected by another object.
Modification Impact
Changes to an instance variable are local to that instance. This dynamic nature allows objects to evolve independently over their lifecycle.
Key Differences Summarized
Scope and Accessibility
- Class variables are shared across all instances, with a global scope within the class context.
- Instance variables are unique to each object, with scope limited to the instance they belong to.
Modification Effects
- Changes to class variables impact all instances of the class.
- Changes to instance variables are isolated to the specific object.
Use Case Scenarios
- Class variables are used for data and behaviors shared by all instances.
- Instance variables are used for data and behaviors unique to each instance.
Examples in Code
Demonstration with Python
Let’s illustrate the differences with a Python example:
pythonCopy code
class MyClass: class_var = 0 # Class variable def __init__(self, instance_var): self.instance_var = instance_var # Instance variable # Creating instances obj1 = MyClass(10) obj2 = MyClass(20) # Accessing class variable print(MyClass.class_var) # Output: 0 print(obj1.class_var) # Output: 0 print(obj2.class_var) # Output: 0 # Modifying class variable MyClass.class_var = 2 print(obj1.class_var) # Output: 2 print(obj2.class_var) # Output: 2 # Accessing and modifying instance variables print(obj1.instance_var) # Output: 10 obj1.instance_var = 30 print(obj1.instance_var) # Output: 30 print(obj2.instance_var) # Output: 20
Comparative Analysis
This example highlights the shared nature of class variables and the independent nature of instance variables. Modifying class_var
through any instance or the class itself reflects across all instances. However, modifying instance_var
in obj1
does not affect instance_var
in obj2
, showcasing their independence.
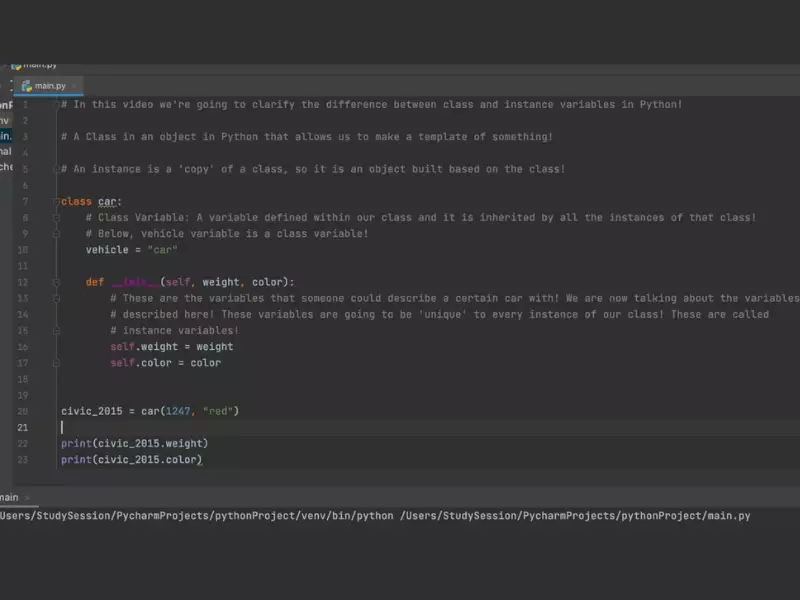
Best Practices for Using Class and Instance Variables
When to Use Class Variables
Class variables serve a unique purpose in object-oriented programming. They are best used when you need a property that is common to all instances of a class. This includes scenarios such as:
- Global constants: Values that do not change and are shared across instances.
- Shared data: Information that needs to be accessible by every instance of a class, like a counter that tracks the number of instances created.
Key Tips:
- Ensure class variables are immutable to prevent accidental changes that affect all instances.
- Use class variables for data that truly needs to be shared to avoid unnecessary dependencies between instances.
When to Use Instance Variables
Instance variables are at the heart of object-oriented programming, allowing for each object to maintain its own state. They are ideal for:
- Storing object-specific data: Attributes that vary from one instance to another.
- Encapsulating state information: Ensuring that an object’s data is hidden and safe from unauthorized access.
Key Tips:
- Initialize instance variables in the constructor to ensure that every object starts with a well-defined state.
- Use instance variables to represent the properties of an object that can change over its lifetime.
Avoiding Common Pitfalls
Both class and instance variables are powerful tools, but their misuse can lead to bugs and inefficient code. Here are some pitfalls to avoid:
- Overuse of class variables: This can lead to difficult-to-track bugs and issues with data integrity.
- Confusion between class and instance variables: Always be clear about whether a variable should be shared among all instances or unique to each instance.
- Not initializing instance variables properly: This can lead to unexpected behavior and errors.
Performance Considerations
Memory Usage
Class and instance variables have different impacts on memory usage. Since class variables are shared across instances, they can be more memory-efficient for data that does not need to be duplicated. On the other hand, each instance variable is unique to an instance, which means more memory is consumed as more instances are created.
Execution Speed
The use of class and instance variables can also affect the execution speed of a program. Accessing class variables might be slightly faster when the data is used frequently across many instances, as there is only one reference to keep track of. However, the difference is often negligible in most applications. The key to optimizing performance lies in the proper use of variables according to their intended purpose rather than in their inherent speed advantages.
Advanced Concepts
Inheritance Implications
Inheritance introduces another layer of complexity to the use of class and instance variables. When a class inherits from another class, it also inherits its class variables. However, how these variables are accessed and modified can have different outcomes:
- Shadowing class variables: A subclass can declare a class variable with the same name as an inherited class variable, effectively shadowing the superclass variable.
- Accessing superclass variables: Subclasses can access and modify superclass class variables, affecting all instances of both the superclass and the subclass.
Overriding Class Variables
While class variables cannot be overridden in the same way that methods can, subclasses can define a class variable with the same name, which can lead to unexpected behavior. This is particularly true in dynamic languages like Python, where the scope of variables is determined at runtime.
Best Practice:
- Avoid redefining class variables in subclasses to maintain clarity and predictability in the codebase.
- Use instance variables or class methods for behavior that needs to be customized in subclasses.
Frequently Asked Questions
What are class variables?
Class variables are variables declared within a class that are shared among all instances of that class. They are used to store values that are constant or common across all objects of the class, providing a centralized place for data that needs to be accessible by all instances.
How do instance variables differ from class variables?
Instance variables are unique to each instance of a class and are used to store the properties and states that define an individual object. Unlike class variables, which are shared and have a static value across instances, instance variables ensure that each object can maintain its own state independently.
When should you use class variables over instance variables?
Class variables should be used when you need to store data that is common to all instances of a class, such as configuration settings or counters that apply to the class as a whole. Instance variables are suitable for data that is specific to an individual object and varies from one instance to another.
Can instance variables override class variables?
Instance variables cannot directly override class variables. However, if an instance variable and a class variable share the same name, the instance variable will shadow the class variable within the scope of that instance, giving precedence to the instance variable.
Conclusion
The distinction between class and instance variables underpins the flexibility and power of object-oriented programming. By judiciously employing these variables, developers can craft robust and scalable software architectures that are capable of handling complex data structures and behaviors. Understanding the differences and appropriate use cases for each type of variable is essential for any software developer looking to build efficient and maintainable code.
In conclusion, the thoughtful application of class and instance variables plays a crucial role in the development of object-oriented software. Their correct usage not only enhances code readability and maintainability but also optimizes memory usage and performance. As such, a deep understanding of these variables and their implications is indispensable for crafting sophisticated and high-performing software solutions.