Python’s dynamic nature allows for efficient and flexible manipulation of data structures, among which lists are the most commonly used. The ability to modify lists dynamically is fundamental to Python programming, allowing developers to handle data more effectively. Two of the most crucial methods for list modification are append
and extend
. Each plays a significant role in how data is added to lists, but they do so in distinct ways that can impact the functionality of a Python script.
The difference between append
and extend
in Python lies in their approach to adding elements to a list. Append
adds its argument as a single element to the end of a list, increasing the list’s length by one. Extend
, on the other hand, iterates over its argument adding each element to the list, thus extending the list by the number of elements in the argument passed to it.
Understanding when to use append
vs. extend
is crucial for efficient Python programming. While both methods aim to add elements to a list, choosing the right one depends on the desired outcome of the list manipulation. This choice can affect the structure of the list, the performance of the program, and ultimately, the successful execution of the task at hand.
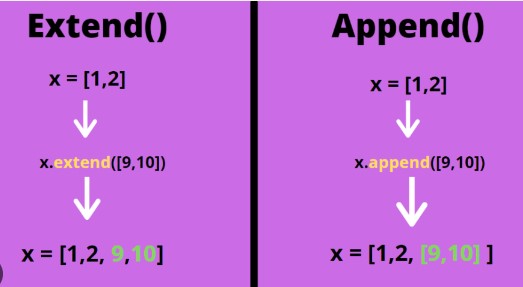
List Basics
Python Lists Explained
In Python, a list is a collection that is ordered and changeable. Lists are one of the most versatile data structures in Python, allowing programmers to store a sequence of items that are accessible via an index. These items can be of any data type, making lists incredibly flexible for various programming needs.
List Creation and Manipulation
Creating a list in Python is straightforward. You simply enclose items within square brackets []
, separating them by commas. For example:
pythonCopy code
my_list = [1, "Hello", 3.14]
Manipulating lists is just as simple. Python offers a variety of methods to modify lists, including adding, removing, or changing items. Two of the primary methods for adding items to a list are append()
and extend()
, which we’ll explore in detail.
The Append Method
Definition and Syntax
The append()
method adds a single item to the end of a list. The syntax is:
pythonCopy code
list.append(item)
Here, list
is your list variable, and item
is what you want to add.
How Append Works
When you use append()
, Python adds the item
to the end of the list, increasing its size by one. Importantly, append()
modifies the original list and does not create a new one.
Use Cases for Append
Use append()
when you need to add a single item to a list. It’s perfect for situations where you’re collecting or accumulating items one at a time. For example, gathering user inputs or during iterations where you add results to a list.
Examples
Adding a number to a list of numbers:
pythonCopy code
numbers = [1, 2, 3] numbers.append(4) print(numbers) # Output: [1, 2, 3, 4]
Appending a list to another list (as a single element):
pythonCopy code
lists = [[1, 2], [3, 4]] lists.append([5, 6]) print(lists) # Output: [[1, 2], [3, 4], [5, 6]]
The Extend Method
Definition and Syntax
The extend()
method adds the elements of an iterable (like a list, tuple, or string) to the end of a list. The syntax is:
pythonCopy code
list.extend(iterable)
iterable
is the collection you want to add to list
.
How Extend Works
Extend()
takes each element from the iterable
and adds it to the list, extending the list’s size by the number of elements in the iterable
. Like append()
, it modifies the original list.
Use Cases for Extend
Use extend()
when you have multiple items to add to a list, especially if these items are already part of another iterable. It’s ideal for concatenating lists or adding multiple elements at once without creating nested lists.
Examples
Extending a list with another list:
pythonCopy code
numbers = [1, 2, 3] more_numbers = [4, 5, 6] numbers.extend(more_numbers) print(numbers) # Output: [1, 2, 3, 4, 5, 6]
Using extend()
with a string:
pythonCopy code
chars = ['a', 'b', 'c'] chars.extend('def') print(chars) # Output: ['a', 'b', 'c', 'd', 'e', 'f']
Key Differences
Comparative Analysis
The main difference between append()
and extend()
in Python lies in how they add elements to a list. While append()
adds its argument as a single element at the end of the list, extend()
unpacks its argument, adding each element to the list. This fundamental distinction affects how you’ll manipulate lists in various scenarios.
Impact on List Size and Structure
- Append: Increases the list size by exactly one, regardless of the argument’s length or type. If you append a list to another list, the entire list is added as a single element, creating a nested list structure.
- Extend: Increases the list size by the number of elements in the iterable passed as an argument. It flattens the structure by adding each element of the iterable to the list, avoiding the creation of nested lists.
Performance Considerations
In terms of performance, extend()
can be more efficient than append()
when you need to add multiple elements. This is because extend()
avoids the overhead associated with repeatedly calling append()
in a loop to add each element of an iterable. However, the performance difference is usually negligible for small lists or few additions.
Practical Applications
When to Use Append
- When adding a single element to a list, regardless of its data type.
- To maintain the integrity of a list or other iterable by adding it as a single, nested element within another list.
- Gathering data incrementally, such as accumulating results in a loop.
When to Use Extend
- When you need to combine two lists or add multiple elements from an iterable to a list.
- To flatten a list of lists or other iterables without introducing nested structures.
- Efficiently adding elements from an iterable without the overhead of looping and appending each one.
Combining Append and Extend in Code
In practice, both append()
and extend()
can be used in conjunction to achieve complex list manipulations. For instance, you might use extend()
to combine several lists into one, then use append()
to add a special marker or separator element at the end. The key is understanding the specific outcome each method offers and selecting the right tool for the job.
Tips and Best Practices
Optimizing List Modifications
- Know Your Data: Choosing between
append()
andextend()
starts with understanding the structure and type of data you’re working with. - Use the Right Tool: For adding single items,
append()
is straightforward and efficient. For adding multiple items from an iterable,extend()
can simplify your code and potentially improve performance. - Preallocate When Possible: If you know the size of the list in advance, preallocating space can improve performance, especially for large lists.
Avoiding Common Mistakes
- Appending Iterables: A common mistake is using
append()
whenextend()
is needed, leading to unexpected nested lists. - Overusing Extend: Conversely, using
extend()
with a non-iterable argument will raise an error. Ensure the argument toextend()
is always an iterable. - Ignoring Return Values: Both
append()
andextend()
modify the list in place and returnNone
. Attempting to use their return value will result in errors or unexpected behavior.
Code Readability and Maintenance
- Explicit is Better Than Implicit: Clearly choosing between
append()
andextend()
makes your intent more understandable to others reading your code. - Commenting: When the choice isn’t obvious, a brief comment explaining why
append()
orextend()
was used can be helpful. - Consistency: Within a project, maintain consistency in how lists are manipulated. This reduces cognitive load and makes the codebase easier to maintain.
FAQs
How do append
and extend
affect list size?
Append
adds its argument as a single element to the end of a list, increasing the list size by one. In contrast, extend
adds each element of its iterable argument to the list, increasing the list size by the number of elements in the iterable. The choice between them depends on whether you want to incorporate a single item or multiple items.
Can I use append
with another list?
Yes, you can use append
with another list. When you do so, the entire list is added as a single element, making it a nested list within the original list. This method is useful when you want to maintain the integrity of the appended list as a distinct item within the original list.
Is it possible to extend a list with non-iterable items?
No, you cannot extend a list with non-iterable items. The extend
method requires an iterable object (like a list, tuple, or string) as its argument. Attempting to extend a list with a non-iterable item will result in a TypeError.
What happens if I append an iterable to a list?
If you append an iterable, like another list, to a list, the iterable is added as a single object at the end of the list. This means the entire iterable becomes one element of the list, not extending the list by the individual elements contained within the iterable.
Conclusion
Choosing between append
and extend
when working with Python lists is more than a matter of syntax; it’s about understanding the specific needs of your data structure and the most efficient way to meet those needs. Append
is ideal for adding single items to a list, while extend
is the method of choice for adding multiple elements. Both methods are indispensable tools in a Python developer’s toolkit, facilitating precise and effective data manipulation.
The distinction between append
and extend
underscores the importance of knowing your tools and the nuances of their operation. With this knowledge, developers can write cleaner, more efficient code, ensuring that their applications run smoothly and perform as intended. As we continue to explore Python’s capabilities, appreciating these differences not only enhances our coding practices but also deepens our understanding of the language’s versatility in handling complex data structures.